mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
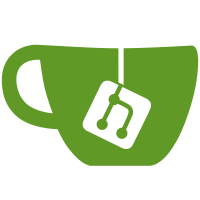
This involved a trivial merge conflict fix in terminal.c because of the way the cherry-pick73b41feba5
differed from its originalbdbd5f429c
. But a more significant rework was needed in windows/console.c, because the updates to confirm_weak_* conflicted with the changes on main to abstract out the ConsoleIO system.
90 lines
2.0 KiB
C
90 lines
2.0 KiB
C
/*
|
|
* Common pieces between the platform console frontend modules.
|
|
*/
|
|
|
|
#include <stdbool.h>
|
|
#include <stdarg.h>
|
|
|
|
#include "putty.h"
|
|
#include "misc.h"
|
|
#include "console.h"
|
|
|
|
const char console_abandoned_msg[] = "Connection abandoned.\n";
|
|
|
|
const SeatDialogPromptDescriptions *console_prompt_descriptions(Seat *seat)
|
|
{
|
|
static const SeatDialogPromptDescriptions descs = {
|
|
.hk_accept_action = "enter \"y\"",
|
|
.hk_connect_once_action = "enter \"n\"",
|
|
.hk_cancel_action = "press Return",
|
|
.hk_cancel_action_Participle = "Pressing Return",
|
|
.weak_accept_action = "enter \"y\"",
|
|
.weak_cancel_action = "enter \"n\"",
|
|
};
|
|
return &descs;
|
|
}
|
|
|
|
bool console_batch_mode = false;
|
|
|
|
bool console_set_batch_mode(bool newvalue)
|
|
{
|
|
console_batch_mode = newvalue;
|
|
return true;
|
|
}
|
|
|
|
/*
|
|
* Error message and/or fatal exit functions, all based on
|
|
* console_print_error_msg which the platform front end provides.
|
|
*/
|
|
void console_print_error_msg_fmt_v(
|
|
const char *prefix, const char *fmt, va_list ap)
|
|
{
|
|
char *msg = dupvprintf(fmt, ap);
|
|
console_print_error_msg(prefix, msg);
|
|
sfree(msg);
|
|
}
|
|
|
|
void console_print_error_msg_fmt(const char *prefix, const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
va_start(ap, fmt);
|
|
console_print_error_msg_fmt_v(prefix, fmt, ap);
|
|
va_end(ap);
|
|
}
|
|
|
|
void modalfatalbox(const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
va_start(ap, fmt);
|
|
console_print_error_msg_fmt_v("FATAL ERROR", fmt, ap);
|
|
va_end(ap);
|
|
cleanup_exit(1);
|
|
}
|
|
|
|
void nonfatal(const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
va_start(ap, fmt);
|
|
console_print_error_msg_fmt_v("ERROR", fmt, ap);
|
|
va_end(ap);
|
|
}
|
|
|
|
void console_connection_fatal(Seat *seat, const char *msg)
|
|
{
|
|
console_print_error_msg("FATAL ERROR", msg);
|
|
cleanup_exit(1);
|
|
}
|
|
|
|
void console_nonfatal(Seat *seat, const char *msg)
|
|
{
|
|
console_print_error_msg("ERROR", msg);
|
|
}
|
|
|
|
/*
|
|
* Console front ends redo their select() or equivalent every time, so
|
|
* they don't need separate timer handling.
|
|
*/
|
|
void timer_change_notify(unsigned long next)
|
|
{
|
|
}
|