mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
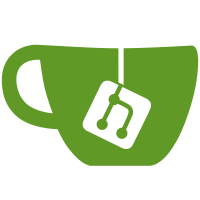
This begins the process of enabling our Windows applications to handle Unicode characters on their command lines which don't fit in the system code page. Instead of passing plain strings to cmdline_process_param, we now pass a partially opaque and platform-specific thing called a CmdlineArg. This has a method that extracts the argument word as a default-encoded string, and another one that tries to extract it as UTF-8 (though it may fail if the UTF-8 isn't available). On Windows, the command line is now constructed by calling split_into_argv_w on the Unicode command line returned by GetCommandLineW(), and the UTF-8 method returns text converted directly from that wide-character form, not going via the system code page. So it _can_ include UTF-8 characters that wouldn't have round-tripped via CP_ACP. This commit introduces the abstraction and switches over the cross-platform and Windows argv-handling code to use it, with minimal functional change. Nothing yet tries to call cmdline_arg_get_utf8(). I say 'cross-platform and Windows' because on the Unix side there's still a lot of use of plain old argv which I haven't converted. That would be a much larger project, and isn't currently needed: the _current_ aim of this abstraction is to get the right things to happen relating to Unicode on Windows, so for code that doesn't run on Windows anyway, it's not adding value. (Also there's a tension with GTK, which wants to talk to standard argv and extract arguments _it_ knows about, so at the very least we'd have to let it munge argv before importing it into this new system.)
30 lines
868 B
C
30 lines
868 B
C
typedef struct psocks_state psocks_state;
|
|
|
|
typedef struct PsocksPlatform PsocksPlatform;
|
|
typedef struct PsocksDataSink PsocksDataSink;
|
|
|
|
/* indices into PsocksDataSink arrays */
|
|
typedef enum PsocksDirection { UP, DN } PsocksDirection;
|
|
|
|
struct PsocksDataSink {
|
|
void (*free)(PsocksDataSink *);
|
|
BinarySink *s[2];
|
|
};
|
|
static inline void pds_free(PsocksDataSink *pds)
|
|
{ pds->free(pds); }
|
|
|
|
PsocksDataSink *pds_stdio(FILE *fp[2]);
|
|
|
|
struct PsocksPlatform {
|
|
PsocksDataSink *(*open_pipes)(
|
|
const char *cmd, const char *const *direction_args,
|
|
const char *index_arg, char **err);
|
|
void (*found_subcommand)(CmdlineArg *arg);
|
|
void (*start_subcommand)(void);
|
|
};
|
|
|
|
psocks_state *psocks_new(const PsocksPlatform *);
|
|
void psocks_free(psocks_state *ps);
|
|
void psocks_cmdline(psocks_state *ps, CmdlineArgList *arglist);
|
|
void psocks_start(psocks_state *ps);
|