mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
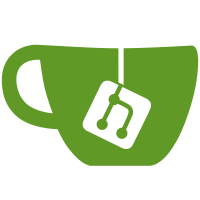
Constructing a FontSpec in platform-independent code is awkward, because you can't call fontspec_new() outside the platform subdirs (since its prototype varies per platform). But sometimes you just need _some_ valid FontSpec, e.g. to put in a Conf that will be used in some place where you don't actually care about font settings, such as a purely CLI program. Both Unix and Windows _have_ an idiom for this, but they're different, because their FontSpec constructors have different prototypes. The existing CLI tools have always had per-platform main source files, so they just use the locally appropriate method of constructing a boring don't-care FontSpec. But if you want a _platform-independent_ main source file, such as you might find in a test program, then that's rather awkward. Better to have a platform-independent API for making a default FontSpec.
41 lines
691 B
C
41 lines
691 B
C
/*
|
|
* Implementation of FontSpec for Unix. This is more or less
|
|
* degenerate - on this platform a font specification is just a
|
|
* string.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
FontSpec *fontspec_new(const char *name)
|
|
{
|
|
FontSpec *f = snew(FontSpec);
|
|
f->name = dupstr(name);
|
|
return f;
|
|
}
|
|
|
|
FontSpec *fontspec_new_default(void)
|
|
{
|
|
return fontspec_new("");
|
|
}
|
|
|
|
FontSpec *fontspec_copy(const FontSpec *f)
|
|
{
|
|
return fontspec_new(f->name);
|
|
}
|
|
|
|
void fontspec_free(FontSpec *f)
|
|
{
|
|
sfree(f->name);
|
|
sfree(f);
|
|
}
|
|
|
|
void fontspec_serialise(BinarySink *bs, FontSpec *f)
|
|
{
|
|
put_asciz(bs, f->name);
|
|
}
|
|
|
|
FontSpec *fontspec_deserialise(BinarySource *src)
|
|
{
|
|
return fontspec_new(get_asciz(src));
|
|
}
|