mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 09:27:59 +00:00
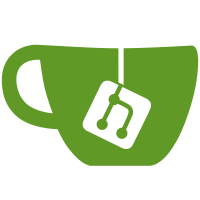
Constructing a FontSpec in platform-independent code is awkward, because you can't call fontspec_new() outside the platform subdirs (since its prototype varies per platform). But sometimes you just need _some_ valid FontSpec, e.g. to put in a Conf that will be used in some place where you don't actually care about font settings, such as a purely CLI program. Both Unix and Windows _have_ an idiom for this, but they're different, because their FontSpec constructors have different prototypes. The existing CLI tools have always had per-platform main source files, so they just use the locally appropriate method of constructing a boring don't-care FontSpec. But if you want a _platform-independent_ main source file, such as you might find in a test program, then that's rather awkward. Better to have a platform-independent API for making a default FontSpec.
49 lines
1019 B
C
49 lines
1019 B
C
/*
|
|
* Implementation of FontSpec for Windows.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
FontSpec *fontspec_new(const char *name, bool bold, int height, int charset)
|
|
{
|
|
FontSpec *f = snew(FontSpec);
|
|
f->name = dupstr(name);
|
|
f->isbold = bold;
|
|
f->height = height;
|
|
f->charset = charset;
|
|
return f;
|
|
}
|
|
|
|
FontSpec *fontspec_new_default(void)
|
|
{
|
|
return fontspec_new("", false, 0, 0);
|
|
}
|
|
|
|
FontSpec *fontspec_copy(const FontSpec *f)
|
|
{
|
|
return fontspec_new(f->name, f->isbold, f->height, f->charset);
|
|
}
|
|
|
|
void fontspec_free(FontSpec *f)
|
|
{
|
|
sfree(f->name);
|
|
sfree(f);
|
|
}
|
|
|
|
void fontspec_serialise(BinarySink *bs, FontSpec *f)
|
|
{
|
|
put_asciz(bs, f->name);
|
|
put_uint32(bs, f->isbold);
|
|
put_uint32(bs, f->height);
|
|
put_uint32(bs, f->charset);
|
|
}
|
|
|
|
FontSpec *fontspec_deserialise(BinarySource *src)
|
|
{
|
|
const char *name = get_asciz(src);
|
|
unsigned isbold = get_uint32(src);
|
|
unsigned height = get_uint32(src);
|
|
unsigned charset = get_uint32(src);
|
|
return fontspec_new(name, isbold, height, charset);
|
|
}
|