mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 01:18:00 +00:00
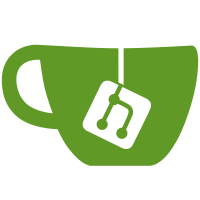
In commitf9e572595b
I claimed that I'd removed very nearly all the global and static variables from windows/window.c. It turns out that this was wildly overoptimistic - I missed quite a few of them! I'm not quite sure how I managed that; my best guess is that I used an underpowered 'nm' command that failed to find some classes of variable. Some of the remaining function-scope statics were removed completely by commitafb3dab1e9
just now. In this commit, I've swept up some more and turn them into fields of WinGuiSeat, where they should have been moved last September. The (hopefully complete this time) list of remaining variables, generated by running this rune in the Windows build directory: nm windows/CMakeFiles/putty.dir/window.c.obj | grep -E '^([^ ]+)? *[bBcCdDgGsS] [^\.]' consists of the following variables which are legitimately global across the whole process and not related to a particular window: - 'hinst' and 'hprev', instance handles for Windows loadable modules - 'classname' in the terminal_window_class_a() and terminal_window_class_w() functions, which allocate a window class reusably - some pointers to Windows API functions retrieved via the DECL_WINDOWS_FUNCTION / GET_WINDOWS_FUNCTION system, such as p_AdjustWindowRectExForDpi and p_FlashWindowEx - some pointers to Windows API functions set up by assigning them at startup to the right one of the ANSI or Unicode version depending on the Windows version, e.g. sw_DefWindowProc and sw_DispatchMessage - 'unicode_window', a boolean flag set at the same time as those sw_Foo function pointers - 'sesslist', storing the last-retrieved version of the saved sessions menu - 'cursor_visible' in show_mouseptr() and 'forced_visible' in update_mouse_pointer(), each of which tracks the cumulative number of times that function has shown or hidden the mouse pointer, so as to manage its effect on the global state updated by ShowCursor - 'trust_icon', loaded from the executable's resources - 'wgslisthead', the list of all active WinGuiSeats - 'wm_mousewheel', the window-message id we use for mouse wheel events and the following which are nothing to do with our code: - '_OptionsStorage' in __local_stdio_printf_options() and __local_stdio_scanf_options(), which I'd never noticed before, but apparently are internal to a standard library header.
166 lines
3.7 KiB
C
166 lines
3.7 KiB
C
/*
|
|
* Main state structure for an instance of the Windows PuTTY front
|
|
* end, containing all the PuTTY objects and all the Windows API
|
|
* resources like the window handle.
|
|
*/
|
|
|
|
typedef struct WinGuiSeat WinGuiSeat;
|
|
|
|
struct PopupMenu {
|
|
HMENU menu;
|
|
};
|
|
enum { SYSMENU, CTXMENU }; /* indices into popup_menus field */
|
|
|
|
#define FONT_NORMAL 0
|
|
#define FONT_BOLD 1
|
|
#define FONT_UNDERLINE 2
|
|
#define FONT_BOLDUND 3
|
|
#define FONT_WIDE 0x04
|
|
#define FONT_HIGH 0x08
|
|
#define FONT_NARROW 0x10
|
|
|
|
#define FONT_OEM 0x20
|
|
#define FONT_OEMBOLD 0x21
|
|
#define FONT_OEMUND 0x22
|
|
#define FONT_OEMBOLDUND 0x23
|
|
|
|
#define FONT_MAXNO 0x40
|
|
#define FONT_SHIFT 5
|
|
|
|
enum BoldMode {
|
|
BOLD_NONE, BOLD_SHADOW, BOLD_FONT
|
|
};
|
|
enum UnderlineMode {
|
|
UND_LINE, UND_FONT
|
|
};
|
|
|
|
struct _dpi_info {
|
|
POINT cur_dpi;
|
|
RECT new_wnd_rect;
|
|
};
|
|
|
|
/*
|
|
* Against the future possibility of having more than one of these
|
|
* in a process (and the current possibility of having zero), we
|
|
* keep a linked list of all live WinGuiSeats, so that cleanups
|
|
* can be done to any that exist.
|
|
*/
|
|
struct WinGuiSeatListNode {
|
|
struct WinGuiSeatListNode *next, *prev;
|
|
};
|
|
extern struct WinGuiSeatListNode wgslisthead; /* static end pointer */
|
|
|
|
struct WinGuiSeat {
|
|
struct WinGuiSeatListNode wgslistnode;
|
|
|
|
Seat seat;
|
|
TermWin termwin;
|
|
LogPolicy logpolicy;
|
|
|
|
HWND term_hwnd;
|
|
|
|
int extra_width, extra_height;
|
|
int font_width, font_height;
|
|
bool font_dualwidth, font_varpitch;
|
|
int offset_width, offset_height;
|
|
bool was_zoomed;
|
|
int prev_rows, prev_cols; // FIXME I don't think these are even used
|
|
|
|
HBITMAP caretbm;
|
|
int caret_x, caret_y;
|
|
|
|
int kbd_codepage;
|
|
|
|
Ldisc *ldisc;
|
|
Backend *backend;
|
|
|
|
cmdline_get_passwd_input_state cmdline_get_passwd_state;
|
|
|
|
struct unicode_data ucsdata;
|
|
bool session_closed;
|
|
bool reconfiguring;
|
|
|
|
const SessionSpecial *specials;
|
|
HMENU specials_menu;
|
|
int n_specials;
|
|
|
|
struct PopupMenu popup_menus[2];
|
|
HMENU savedsess_menu;
|
|
|
|
Conf *conf;
|
|
LogContext *logctx;
|
|
Terminal *term;
|
|
|
|
int cursor_type;
|
|
int vtmode;
|
|
|
|
HFONT fonts[FONT_MAXNO];
|
|
LOGFONT lfont;
|
|
bool fontflag[FONT_MAXNO];
|
|
enum BoldMode bold_font_mode;
|
|
|
|
bool bold_colours;
|
|
enum UnderlineMode und_mode;
|
|
int descent, font_strikethrough_y;
|
|
|
|
COLORREF colours[OSC4_NCOLOURS];
|
|
HPALETTE pal;
|
|
LPLOGPALETTE logpal;
|
|
bool tried_pal;
|
|
COLORREF colorref_modifier;
|
|
|
|
struct _dpi_info dpi_info;
|
|
|
|
int dbltime, lasttime, lastact;
|
|
Mouse_Button lastbtn;
|
|
|
|
bool send_raw_mouse;
|
|
int wheel_accumulator;
|
|
|
|
bool pointer_indicates_raw_mouse;
|
|
|
|
BusyStatus busy_status;
|
|
|
|
wchar_t *window_name, *icon_name;
|
|
|
|
int alt_numberpad_accumulator;
|
|
int compose_state;
|
|
int compose_char;
|
|
WPARAM compose_keycode;
|
|
|
|
HDC wintw_hdc;
|
|
|
|
bool resizing;
|
|
bool need_backend_resize;
|
|
|
|
long next_flash;
|
|
bool flashing;
|
|
|
|
long last_beep_time;
|
|
|
|
bool ignore_clip;
|
|
bool fullscr_on_max;
|
|
bool processed_resize;
|
|
bool in_scrollbar_loop;
|
|
UINT last_mousemove;
|
|
WPARAM last_wm_mousemove_wParam, last_wm_ncmousemove_wParam;
|
|
LPARAM last_wm_mousemove_lParam, last_wm_ncmousemove_lParam;
|
|
wchar_t pending_surrogate;
|
|
};
|
|
|
|
extern const LogPolicyVtable win_gui_logpolicy_vt; /* in dialog.c */
|
|
|
|
static inline void wgs_link(WinGuiSeat *wgs)
|
|
{
|
|
wgs->wgslistnode.prev = wgslisthead.prev;
|
|
wgs->wgslistnode.next = &wgslisthead;
|
|
wgs->wgslistnode.prev->next = &wgs->wgslistnode;
|
|
wgs->wgslistnode.next->prev = &wgs->wgslistnode;
|
|
}
|
|
|
|
static inline void wgs_unlink(WinGuiSeat *wgs)
|
|
{
|
|
wgs->wgslistnode.prev->next = wgs->wgslistnode.next;
|
|
wgs->wgslistnode.next->prev = wgs->wgslistnode.prev;
|
|
}
|