mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-04-03 20:20:13 -05:00
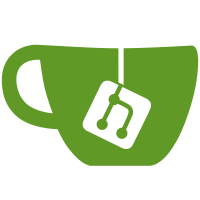
It's had its day. It was there to support pre-WinNT platforms, on which the security APIs don't exist - but more specifically, it was there to support _build tools_ that only knew about pre-WinNT versions of Windows, so that you couldn't even compile a program that would _try_ to refer to the interprocess security APIs. But we don't support those build systems any more in any case: more recent changes like the assumption of (most of) C99 will have stopped this code from building with compilers that old. So there's no reason to clutter the code with backwards compatibility features that won't help. I left NO_SECURITY in place during the CMake migration, so that _just_ in case it needs resurrecting, some version of it will be available in the git history. But I don't expect it to be needed, and I'm deleting the whole thing now. The _runtime_ check for interprocess security libraries is still in place. So PuTTY tools built with a modern toolchain can still at least try to run on the Win95/98/ME series, and they should detect that those system DLLs don't exist and proceed sensibly in their absence. That may also be a thing to throw out sooner or later, but I haven't thrown it out as part of this commit.
86 lines
2.4 KiB
C
86 lines
2.4 KiB
C
/*
|
|
* wincapi.c: implementation of wincapi.h.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
#include "putty.h"
|
|
#include "ssh.h"
|
|
|
|
#include "wincapi.h"
|
|
|
|
DEF_WINDOWS_FUNCTION(CryptProtectMemory);
|
|
|
|
bool got_crypt(void)
|
|
{
|
|
static bool attempted = false;
|
|
static bool successful;
|
|
static HMODULE crypt;
|
|
|
|
if (!attempted) {
|
|
attempted = true;
|
|
crypt = load_system32_dll("crypt32.dll");
|
|
successful = crypt &&
|
|
GET_WINDOWS_FUNCTION(crypt, CryptProtectMemory);
|
|
}
|
|
return successful;
|
|
}
|
|
|
|
char *capi_obfuscate_string(const char *realname)
|
|
{
|
|
char *cryptdata;
|
|
int cryptlen;
|
|
unsigned char digest[32];
|
|
char retbuf[65];
|
|
int i;
|
|
|
|
cryptlen = strlen(realname) + 1;
|
|
cryptlen += CRYPTPROTECTMEMORY_BLOCK_SIZE - 1;
|
|
cryptlen /= CRYPTPROTECTMEMORY_BLOCK_SIZE;
|
|
cryptlen *= CRYPTPROTECTMEMORY_BLOCK_SIZE;
|
|
|
|
cryptdata = snewn(cryptlen, char);
|
|
memset(cryptdata, 0, cryptlen);
|
|
strcpy(cryptdata, realname);
|
|
|
|
/*
|
|
* CRYPTPROTECTMEMORY_CROSS_PROCESS causes CryptProtectMemory to
|
|
* use the same key in all processes with this user id, meaning
|
|
* that the next PuTTY process calling this function with the same
|
|
* input will get the same data.
|
|
*
|
|
* (Contrast with CryptProtectData, which invents a new session
|
|
* key every time since its API permits returning more data than
|
|
* was input, so calling _that_ and hashing the output would not
|
|
* be stable.)
|
|
*
|
|
* We don't worry too much if this doesn't work for some reason.
|
|
* Omitting this step still has _some_ privacy value (in that
|
|
* another user can test-hash things to confirm guesses as to
|
|
* where you might be connecting to, but cannot invert SHA-256 in
|
|
* the absence of any plausible guess). So we don't abort if we
|
|
* can't call CryptProtectMemory at all, or if it fails.
|
|
*/
|
|
if (got_crypt())
|
|
p_CryptProtectMemory(cryptdata, cryptlen,
|
|
CRYPTPROTECTMEMORY_CROSS_PROCESS);
|
|
|
|
/*
|
|
* We don't want to give away the length of the hostname either,
|
|
* so having got it back out of CryptProtectMemory we now hash it.
|
|
*/
|
|
hash_simple(&ssh_sha256, make_ptrlen(cryptdata, cryptlen), digest);
|
|
|
|
sfree(cryptdata);
|
|
|
|
/*
|
|
* Finally, make printable.
|
|
*/
|
|
for (i = 0; i < 32; i++) {
|
|
sprintf(retbuf + 2*i, "%02x", digest[i]);
|
|
/* the last of those will also write the trailing NUL */
|
|
}
|
|
|
|
return dupstr(retbuf);
|
|
}
|