mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-04-03 20:20:13 -05:00
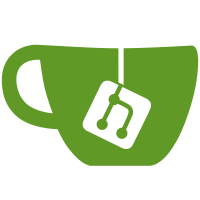
The refactored sshaes.c gives me a convenient slot to drop in a second hardware-accelerated AES implementation, similar to the existing one but using Arm NEON intrinsics in place of the x86 AES-NI ones. This needed a minor structural change, because Arm systems are often heterogeneous, containing more than one type of CPU which won't necessarily all support the same set of architecture features. So you can't test at run time for the presence of AES acceleration by querying the CPU you're running on - even if you found a way to do it, the answer wouldn't be reliable once the OS started migrating your process between CPUs. Instead, you have to ask the OS itself, because only that knows about _all_ the CPUs on the system. So that means the aes_hw_available() mechanism has to extend a tentacle into each platform subdirectory. The trickiest part was the nest of ifdefs that tries to detect whether the compiler can support the necessary parts. I had successful test-compiles on several compilers, and was able to run the code directly on an AArch64 tablet (so I know it passes cryptsuite), but it's likely that at least some Arm platforms won't be able to build it because of some path through the ifdefs that I haven't been able to test yet.
27 lines
415 B
C
27 lines
415 B
C
#include "ssh.h"
|
|
|
|
#if defined __linux__ && (defined __arm__ || defined __aarch64__)
|
|
|
|
#include <sys/auxv.h>
|
|
#include <asm/hwcap.h>
|
|
|
|
bool platform_aes_hw_available(void)
|
|
{
|
|
#if defined HWCAP_AES
|
|
return getauxval(AT_HWCAP) & HWCAP_AES;
|
|
#elif defined HWCAP2_AES
|
|
return getauxval(AT_HWCAP2) & HWCAP2_AES;
|
|
#else
|
|
return false;
|
|
#endif
|
|
}
|
|
|
|
#else
|
|
|
|
bool platform_aes_hw_available(void)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
#endif
|