mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-25 01:02:24 +00:00
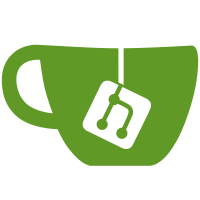
This notifies the Seat that the entire backend session has finished and closed its network connection - or rather, that it _might_ have done, and that the frontend should check backend_connected() if it wasn't planning to do so already. The existing Seat implementations haven't needed this: the GUI ones don't actually need to do anything specific when the network connection goes away, and the CLI ones deal with it by being in charge of their own event loop so that they can easily check backend_connected() at every possible opportunity in any case. But I'm about to introduce a new Seat implementation that does need to know this, and doesn't have any other way to get notified of it.
44 lines
2.2 KiB
C
44 lines
2.2 KiB
C
/*
|
|
* Stub methods usable by Seat implementations.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
size_t nullseat_output(
|
|
Seat *seat, bool is_stderr, const void *data, size_t len) { return 0; }
|
|
bool nullseat_eof(Seat *seat) { return true; }
|
|
int nullseat_get_userpass_input(
|
|
Seat *seat, prompts_t *p, bufchain *input) { return 0; }
|
|
void nullseat_notify_remote_exit(Seat *seat) {}
|
|
void nullseat_notify_remote_disconnect(Seat *seat) {}
|
|
void nullseat_connection_fatal(Seat *seat, const char *message) {}
|
|
void nullseat_update_specials_menu(Seat *seat) {}
|
|
char *nullseat_get_ttymode(Seat *seat, const char *mode) { return NULL; }
|
|
void nullseat_set_busy_status(Seat *seat, BusyStatus status) {}
|
|
int nullseat_verify_ssh_host_key(
|
|
Seat *seat, const char *host, int port, const char *keytype,
|
|
char *keystr, const char *keydisp, char **key_fingerprints,
|
|
void (*callback)(void *ctx, int result), void *ctx) { return 0; }
|
|
int nullseat_confirm_weak_crypto_primitive(
|
|
Seat *seat, const char *algtype, const char *algname,
|
|
void (*callback)(void *ctx, int result), void *ctx) { return 0; }
|
|
int nullseat_confirm_weak_cached_hostkey(
|
|
Seat *seat, const char *algname, const char *betteralgs,
|
|
void (*callback)(void *ctx, int result), void *ctx) { return 0; }
|
|
bool nullseat_is_never_utf8(Seat *seat) { return false; }
|
|
bool nullseat_is_always_utf8(Seat *seat) { return true; }
|
|
void nullseat_echoedit_update(Seat *seat, bool echoing, bool editing) {}
|
|
const char *nullseat_get_x_display(Seat *seat) { return NULL; }
|
|
bool nullseat_get_windowid(Seat *seat, long *id_out) { return false; }
|
|
bool nullseat_get_window_pixel_size(
|
|
Seat *seat, int *width, int *height) { return false; }
|
|
StripCtrlChars *nullseat_stripctrl_new(
|
|
Seat *seat, BinarySink *bs_out, SeatInteractionContext sic) {return NULL;}
|
|
bool nullseat_set_trust_status(Seat *seat, bool tr) { return false; }
|
|
bool nullseat_set_trust_status_vacuously(Seat *seat, bool tr) { return true; }
|
|
bool nullseat_verbose_no(Seat *seat) { return false; }
|
|
bool nullseat_verbose_yes(Seat *seat) { return true; }
|
|
bool nullseat_interactive_no(Seat *seat) { return false; }
|
|
bool nullseat_interactive_yes(Seat *seat) { return true; }
|
|
bool nullseat_get_cursor_position(Seat *seat, int *x, int *y) { return false; }
|