mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-10 01:48:00 +00:00
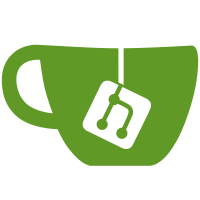
This parameter was undocumented, and Windows-specific: its semantics date from before PuTTY was cross-platform, and are "Pass this flags parameter straight through to the Win32 API's conversion functions". So in Windows platform code you can pass flags like MB_USEGLYPHCHARS, but in cross-platform code, you dare not pass anything nonzero at all because the Unix frontend won't recognise it (or, likely, even compile). I've kept the flag for now in the underlying mb_to_wc / wc_to_mb functions. Partly that's because there's one place in the Windows code where the parameter _is_ used; mostly, it's because I'm about to replace those functions anyway, so there's no point in editing all the call sites twice.
93 lines
2.0 KiB
C
93 lines
2.0 KiB
C
/*
|
|
* Implementation of Filename for Windows.
|
|
*/
|
|
|
|
#include <wchar.h>
|
|
|
|
#include "putty.h"
|
|
|
|
Filename *filename_from_str(const char *str)
|
|
{
|
|
Filename *fn = snew(Filename);
|
|
fn->cpath = dupstr(str);
|
|
fn->wpath = dup_mb_to_wc(DEFAULT_CODEPAGE, fn->cpath);
|
|
fn->utf8path = encode_wide_string_as_utf8(fn->wpath);
|
|
return fn;
|
|
}
|
|
|
|
Filename *filename_from_wstr(const wchar_t *str)
|
|
{
|
|
Filename *fn = snew(Filename);
|
|
fn->wpath = dupwcs(str);
|
|
fn->cpath = dup_wc_to_mb(DEFAULT_CODEPAGE, fn->wpath, "?");
|
|
fn->utf8path = encode_wide_string_as_utf8(fn->wpath);
|
|
return fn;
|
|
}
|
|
|
|
Filename *filename_from_utf8(const char *ustr)
|
|
{
|
|
Filename *fn = snew(Filename);
|
|
fn->utf8path = dupstr(ustr);
|
|
fn->wpath = decode_utf8_to_wide_string(fn->utf8path);
|
|
fn->cpath = dup_wc_to_mb(DEFAULT_CODEPAGE, fn->wpath, "?");
|
|
return fn;
|
|
}
|
|
|
|
Filename *filename_copy(const Filename *fn)
|
|
{
|
|
Filename *newfn = snew(Filename);
|
|
newfn->cpath = dupstr(fn->cpath);
|
|
newfn->wpath = dupwcs(fn->wpath);
|
|
newfn->utf8path = dupstr(fn->utf8path);
|
|
return newfn;
|
|
}
|
|
|
|
const char *filename_to_str(const Filename *fn)
|
|
{
|
|
return fn->cpath; /* FIXME */
|
|
}
|
|
|
|
bool filename_equal(const Filename *f1, const Filename *f2)
|
|
{
|
|
/* wpath is primary: two filenames refer to the same file if they
|
|
* have the same wpath */
|
|
return !wcscmp(f1->wpath, f2->wpath);
|
|
}
|
|
|
|
bool filename_is_null(const Filename *fn)
|
|
{
|
|
return !*fn->wpath;
|
|
}
|
|
|
|
void filename_free(Filename *fn)
|
|
{
|
|
sfree(fn->wpath);
|
|
sfree(fn->cpath);
|
|
sfree(fn->utf8path);
|
|
sfree(fn);
|
|
}
|
|
|
|
void filename_serialise(BinarySink *bs, const Filename *f)
|
|
{
|
|
put_asciz(bs, f->utf8path);
|
|
}
|
|
Filename *filename_deserialise(BinarySource *src)
|
|
{
|
|
const char *utf8 = get_asciz(src);
|
|
return filename_from_utf8(utf8);
|
|
}
|
|
|
|
char filename_char_sanitise(char c)
|
|
{
|
|
if (strchr("<>:\"/\\|?*", c))
|
|
return '.';
|
|
return c;
|
|
}
|
|
|
|
FILE *f_open(const Filename *fn, const char *mode, bool isprivate)
|
|
{
|
|
wchar_t *wmode = dup_mb_to_wc(DEFAULT_CODEPAGE, mode);
|
|
return _wfopen(fn->wpath, wmode);
|
|
sfree(wmode);
|
|
}
|