mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
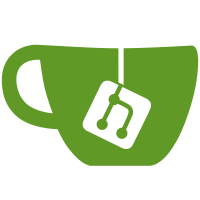
Now when we construct a packet containing sensitive data, we just set a field saying '... and make it take up at least this much space, to disguise its true size', and nothing in the rest of the system worries about that flag until ssh2bpp.c acts on it. Also, I've changed the strategy for doing the padding. Previously, we were following the real packet with an SSH_MSG_IGNORE to make up the size. But that was only a partial defence: it works OK against passive traffic analysis, but an attacker proxying the TCP stream and dribbling it out one byte at a time could still have found out the size of the real packet by noting when the dribbled data provoked a response. Now I put the SSH_MSG_IGNORE _first_, which should defeat that attack. But that in turn doesn't work when we're doing compression, because we can't predict the compressed sizes accurately enough to make that strategy sensible. Fortunately, compression provides an alternative strategy anyway: if we've got zlib turned on when we send one of these sensitive packets, then we can pad out the compressed zlib data as much as we like by adding empty RFC1951 blocks (effectively chaining ZLIB_PARTIAL_FLUSHes). So both strategies should now be dribble-proof.
54 lines
1.7 KiB
C
54 lines
1.7 KiB
C
/*
|
|
* Abstraction of the binary packet protocols used in SSH.
|
|
*/
|
|
|
|
#ifndef PUTTY_SSHBPP_H
|
|
#define PUTTY_SSHBPP_H
|
|
|
|
typedef struct BinaryPacketProtocol BinaryPacketProtocol;
|
|
|
|
struct BinaryPacketProtocolVtable {
|
|
void (*free)(BinaryPacketProtocol *);
|
|
void (*handle_input)(BinaryPacketProtocol *);
|
|
PktOut *(*new_pktout)(int type);
|
|
void (*format_packet)(BinaryPacketProtocol *, PktOut *);
|
|
};
|
|
|
|
struct BinaryPacketProtocol {
|
|
const struct BinaryPacketProtocolVtable *vt;
|
|
bufchain *in_raw, *out_raw;
|
|
PacketQueue *in_pq;
|
|
PacketLogSettings *pls;
|
|
void *logctx;
|
|
|
|
int seen_disconnect;
|
|
char *error;
|
|
};
|
|
|
|
#define ssh_bpp_free(bpp) ((bpp)->vt->free(bpp))
|
|
#define ssh_bpp_handle_input(bpp) ((bpp)->vt->handle_input(bpp))
|
|
#define ssh_bpp_new_pktout(bpp, type) ((bpp)->vt->new_pktout(type))
|
|
#define ssh_bpp_format_packet(bpp, pkt) ((bpp)->vt->format_packet(bpp, pkt))
|
|
|
|
BinaryPacketProtocol *ssh1_bpp_new(void);
|
|
void ssh1_bpp_new_cipher(BinaryPacketProtocol *bpp,
|
|
const struct ssh_cipher *cipher,
|
|
const void *session_key);
|
|
void ssh1_bpp_start_compression(BinaryPacketProtocol *bpp);
|
|
|
|
BinaryPacketProtocol *ssh2_bpp_new(void);
|
|
void ssh2_bpp_new_outgoing_crypto(
|
|
BinaryPacketProtocol *bpp,
|
|
const struct ssh2_cipher *cipher, const void *ckey, const void *iv,
|
|
const struct ssh_mac *mac, int etm_mode, const void *mac_key,
|
|
const struct ssh_compress *compression);
|
|
void ssh2_bpp_new_incoming_crypto(
|
|
BinaryPacketProtocol *bpp,
|
|
const struct ssh2_cipher *cipher, const void *ckey, const void *iv,
|
|
const struct ssh_mac *mac, int etm_mode, const void *mac_key,
|
|
const struct ssh_compress *compression);
|
|
|
|
BinaryPacketProtocol *ssh2_bare_bpp_new(void);
|
|
|
|
#endif /* PUTTY_SSHBPP_H */
|