mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-02 20:12:48 -05:00
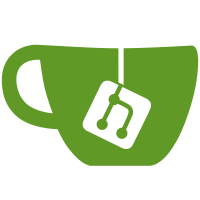
Now I've got FROMFIELD, I can rework it so that structures providing an implementation of the Socket or Plug trait no longer have to have the vtable pointer as the very first thing in the structure. In particular, this means that the ProxySocket structure can now directly implement _both_ the Socket and Plug traits, which is always _logically_ how it's worked, but previously it had to be implemented via two separate structs linked to each other.
68 lines
1.3 KiB
C
68 lines
1.3 KiB
C
/*
|
|
* A dummy Socket implementation which just holds an error message.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <assert.h>
|
|
|
|
#define DEFINE_PLUG_METHOD_MACROS
|
|
#include "tree234.h"
|
|
#include "putty.h"
|
|
#include "network.h"
|
|
|
|
typedef struct {
|
|
char *error;
|
|
Plug plug;
|
|
|
|
const Socket_vtable *sockvt;
|
|
} ErrorSocket;
|
|
|
|
static Plug sk_error_plug(Socket s, Plug p)
|
|
{
|
|
ErrorSocket *es = FROMFIELD(s, ErrorSocket, sockvt);
|
|
Plug ret = es->plug;
|
|
if (p)
|
|
es->plug = p;
|
|
return ret;
|
|
}
|
|
|
|
static void sk_error_close(Socket s)
|
|
{
|
|
ErrorSocket *es = FROMFIELD(s, ErrorSocket, sockvt);
|
|
|
|
sfree(es->error);
|
|
sfree(es);
|
|
}
|
|
|
|
static const char *sk_error_socket_error(Socket s)
|
|
{
|
|
ErrorSocket *es = FROMFIELD(s, ErrorSocket, sockvt);
|
|
return es->error;
|
|
}
|
|
|
|
static char *sk_error_peer_info(Socket s)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static const Socket_vtable ErrorSocket_sockvt = {
|
|
sk_error_plug,
|
|
sk_error_close,
|
|
NULL /* write */,
|
|
NULL /* write_oob */,
|
|
NULL /* write_eof */,
|
|
NULL /* flush */,
|
|
NULL /* set_frozen */,
|
|
sk_error_socket_error,
|
|
sk_error_peer_info,
|
|
};
|
|
|
|
Socket new_error_socket(const char *errmsg, Plug plug)
|
|
{
|
|
ErrorSocket *es = snew(ErrorSocket);
|
|
es->sockvt = &ErrorSocket_sockvt;
|
|
es->plug = plug;
|
|
es->error = dupstr(errmsg);
|
|
return &es->sockvt;
|
|
}
|