mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-10 01:48:00 +00:00
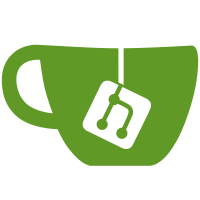
My normal habit these days, in new code, is to treat int and bool as _almost_ completely separate types. I'm still willing to use C's implicit test for zero on an integer (e.g. 'if (!blob.len)' is fine, no need to spell it out as blob.len != 0), but generally, if a variable is going to be conceptually a boolean, I like to declare it bool and assign to it using 'true' or 'false' rather than 0 or 1. PuTTY is an exception, because it predates the C99 bool, and I've stuck to its existing coding style even when adding new code to it. But it's been annoying me more and more, so now that I've decided C99 bool is an acceptable thing to require from our toolchain in the first place, here's a quite thorough trawl through the source doing 'boolification'. Many variables and function parameters are now typed as bool rather than int; many assignments of 0 or 1 to those variables are now spelled 'true' or 'false'. I managed this thorough conversion with the help of a custom clang plugin that I wrote to trawl the AST and apply heuristics to point out where things might want changing. So I've even managed to do a decent job on parts of the code I haven't looked at in years! To make the plugin's work easier, I pushed platform front ends generally in the direction of using standard 'bool' in preference to platform-specific boolean types like Windows BOOL or GTK's gboolean; I've left the platform booleans in places they _have_ to be for the platform APIs to work right, but variables only used by my own code have been converted wherever I found them. In a few places there are int values that look very like booleans in _most_ of the places they're used, but have a rarely-used third value, or a distinction between different nonzero values that most users don't care about. In these cases, I've _removed_ uses of 'true' and 'false' for the return values, to emphasise that there's something more subtle going on than a simple boolean answer: - the 'multisel' field in dialog.h's list box structure, for which the GTK front end in particular recognises a difference between 1 and 2 but nearly everything else treats as boolean - the 'urgent' parameter to plug_receive, where 1 vs 2 tells you something about the specific location of the urgent pointer, but most clients only care about 0 vs 'something nonzero' - the return value of wc_match, where -1 indicates a syntax error in the wildcard. - the return values from SSH-1 RSA-key loading functions, which use -1 for 'wrong passphrase' and 0 for all other failures (so any caller which already knows it's not loading an _encrypted private_ key can treat them as boolean) - term->esc_query, and the 'query' parameter in toggle_mode in terminal.c, which _usually_ hold 0 for ESC[123h or 1 for ESC[?123h, but can also hold -1 for some other intervening character that we don't support. In a few places there's an integer that I haven't turned into a bool even though it really _can_ only take values 0 or 1 (and, as above, tried to make the call sites consistent in not calling those values true and false), on the grounds that I thought it would make it more confusing to imply that the 0 value was in some sense 'negative' or bad and the 1 positive or good: - the return value of plug_accepting uses the POSIXish convention of 0=success and nonzero=error; I think if I made it bool then I'd also want to reverse its sense, and that's a job for a separate piece of work. - the 'screen' parameter to lineptr() in terminal.c, where 0 and 1 represent the default and alternate screens. There's no obvious reason why one of those should be considered 'true' or 'positive' or 'success' - they're just indices - so I've left it as int. ssh_scp_recv had particularly confusing semantics for its previous int return value: its call sites used '<= 0' to check for error, but it never actually returned a negative number, just 0 or 1. Now the function and its call sites agree that it's a bool. In a couple of places I've renamed variables called 'ret', because I don't like that name any more - it's unclear whether it means the return value (in preparation) for the _containing_ function or the return value received from a subroutine call, and occasionally I've accidentally used the same variable for both and introduced a bug. So where one of those got in my way, I've renamed it to 'toret' or 'retd' (the latter short for 'returned') in line with my usual modern practice, but I haven't done a thorough job of finding all of them. Finally, one amusing side effect of doing this is that I've had to separate quite a few chained assignments. It used to be perfectly fine to write 'a = b = c = TRUE' when a,b,c were int and TRUE was just a the 'true' defined by stdbool.h, that idiom provokes a warning from gcc: 'suggest parentheses around assignment used as truth value'!
626 lines
18 KiB
C
626 lines
18 KiB
C
/*
|
|
* PLink - a Windows command-line (stdin/stdout) variant of PuTTY.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <assert.h>
|
|
#include <stdarg.h>
|
|
|
|
#define PUTTY_DO_GLOBALS /* actually _define_ globals */
|
|
#include "putty.h"
|
|
#include "storage.h"
|
|
#include "tree234.h"
|
|
#include "winsecur.h"
|
|
|
|
#define WM_AGENT_CALLBACK (WM_APP + 4)
|
|
|
|
struct agent_callback {
|
|
void (*callback)(void *, void *, int);
|
|
void *callback_ctx;
|
|
void *data;
|
|
int len;
|
|
};
|
|
|
|
void cmdline_error(const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
va_start(ap, fmt);
|
|
console_print_error_msg_fmt_v("plink", fmt, ap);
|
|
va_end(ap);
|
|
exit(1);
|
|
}
|
|
|
|
HANDLE inhandle, outhandle, errhandle;
|
|
struct handle *stdin_handle, *stdout_handle, *stderr_handle;
|
|
DWORD orig_console_mode;
|
|
|
|
WSAEVENT netevent;
|
|
|
|
static Backend *backend;
|
|
static Conf *conf;
|
|
|
|
bool term_ldisc(Terminal *term, int mode)
|
|
{
|
|
return false;
|
|
}
|
|
static void plink_echoedit_update(Seat *seat, bool echo, bool edit)
|
|
{
|
|
/* Update stdin read mode to reflect changes in line discipline. */
|
|
DWORD mode;
|
|
|
|
mode = ENABLE_PROCESSED_INPUT;
|
|
if (echo)
|
|
mode = mode | ENABLE_ECHO_INPUT;
|
|
else
|
|
mode = mode & ~ENABLE_ECHO_INPUT;
|
|
if (edit)
|
|
mode = mode | ENABLE_LINE_INPUT;
|
|
else
|
|
mode = mode & ~ENABLE_LINE_INPUT;
|
|
SetConsoleMode(inhandle, mode);
|
|
}
|
|
|
|
static int plink_output(Seat *seat, bool is_stderr, const void *data, int len)
|
|
{
|
|
if (is_stderr) {
|
|
handle_write(stderr_handle, data, len);
|
|
} else {
|
|
handle_write(stdout_handle, data, len);
|
|
}
|
|
|
|
return handle_backlog(stdout_handle) + handle_backlog(stderr_handle);
|
|
}
|
|
|
|
static bool plink_eof(Seat *seat)
|
|
{
|
|
handle_write_eof(stdout_handle);
|
|
return false; /* do not respond to incoming EOF with outgoing */
|
|
}
|
|
|
|
static int plink_get_userpass_input(Seat *seat, prompts_t *p, bufchain *input)
|
|
{
|
|
int ret;
|
|
ret = cmdline_get_passwd_input(p);
|
|
if (ret == -1)
|
|
ret = console_get_userpass_input(p);
|
|
return ret;
|
|
}
|
|
|
|
static const SeatVtable plink_seat_vt = {
|
|
plink_output,
|
|
plink_eof,
|
|
plink_get_userpass_input,
|
|
nullseat_notify_remote_exit,
|
|
console_connection_fatal,
|
|
nullseat_update_specials_menu,
|
|
nullseat_get_ttymode,
|
|
nullseat_set_busy_status,
|
|
console_verify_ssh_host_key,
|
|
console_confirm_weak_crypto_primitive,
|
|
console_confirm_weak_cached_hostkey,
|
|
nullseat_is_never_utf8,
|
|
plink_echoedit_update,
|
|
nullseat_get_x_display,
|
|
nullseat_get_windowid,
|
|
nullseat_get_window_pixel_size,
|
|
};
|
|
static Seat plink_seat[1] = {{ &plink_seat_vt }};
|
|
|
|
static DWORD main_thread_id;
|
|
|
|
void agent_schedule_callback(void (*callback)(void *, void *, int),
|
|
void *callback_ctx, void *data, int len)
|
|
{
|
|
struct agent_callback *c = snew(struct agent_callback);
|
|
c->callback = callback;
|
|
c->callback_ctx = callback_ctx;
|
|
c->data = data;
|
|
c->len = len;
|
|
PostThreadMessage(main_thread_id, WM_AGENT_CALLBACK, 0, (LPARAM)c);
|
|
}
|
|
|
|
/*
|
|
* Short description of parameters.
|
|
*/
|
|
static void usage(void)
|
|
{
|
|
printf("Plink: command-line connection utility\n");
|
|
printf("%s\n", ver);
|
|
printf("Usage: plink [options] [user@]host [command]\n");
|
|
printf(" (\"host\" can also be a PuTTY saved session name)\n");
|
|
printf("Options:\n");
|
|
printf(" -V print version information and exit\n");
|
|
printf(" -pgpfp print PGP key fingerprints and exit\n");
|
|
printf(" -v show verbose messages\n");
|
|
printf(" -load sessname Load settings from saved session\n");
|
|
printf(" -ssh -telnet -rlogin -raw -serial\n");
|
|
printf(" force use of a particular protocol\n");
|
|
printf(" -P port connect to specified port\n");
|
|
printf(" -l user connect with specified username\n");
|
|
printf(" -batch disable all interactive prompts\n");
|
|
printf(" -proxycmd command\n");
|
|
printf(" use 'command' as local proxy\n");
|
|
printf(" -sercfg configuration-string (e.g. 19200,8,n,1,X)\n");
|
|
printf(" Specify the serial configuration (serial only)\n");
|
|
printf("The following options only apply to SSH connections:\n");
|
|
printf(" -pw passw login with specified password\n");
|
|
printf(" -D [listen-IP:]listen-port\n");
|
|
printf(" Dynamic SOCKS-based port forwarding\n");
|
|
printf(" -L [listen-IP:]listen-port:host:port\n");
|
|
printf(" Forward local port to remote address\n");
|
|
printf(" -R [listen-IP:]listen-port:host:port\n");
|
|
printf(" Forward remote port to local address\n");
|
|
printf(" -X -x enable / disable X11 forwarding\n");
|
|
printf(" -A -a enable / disable agent forwarding\n");
|
|
printf(" -t -T enable / disable pty allocation\n");
|
|
printf(" -1 -2 force use of particular protocol version\n");
|
|
printf(" -4 -6 force use of IPv4 or IPv6\n");
|
|
printf(" -C enable compression\n");
|
|
printf(" -i key private key file for user authentication\n");
|
|
printf(" -noagent disable use of Pageant\n");
|
|
printf(" -agent enable use of Pageant\n");
|
|
printf(" -noshare disable use of connection sharing\n");
|
|
printf(" -share enable use of connection sharing\n");
|
|
printf(" -hostkey aa:bb:cc:...\n");
|
|
printf(" manually specify a host key (may be repeated)\n");
|
|
printf(" -m file read remote command(s) from file\n");
|
|
printf(" -s remote command is an SSH subsystem (SSH-2 only)\n");
|
|
printf(" -N don't start a shell/command (SSH-2 only)\n");
|
|
printf(" -nc host:port\n");
|
|
printf(" open tunnel in place of session (SSH-2 only)\n");
|
|
printf(" -sshlog file\n");
|
|
printf(" -sshrawlog file\n");
|
|
printf(" log protocol details to a file\n");
|
|
printf(" -shareexists\n");
|
|
printf(" test whether a connection-sharing upstream exists\n");
|
|
exit(1);
|
|
}
|
|
|
|
static void version(void)
|
|
{
|
|
char *buildinfo_text = buildinfo("\n");
|
|
printf("plink: %s\n%s\n", ver, buildinfo_text);
|
|
sfree(buildinfo_text);
|
|
exit(0);
|
|
}
|
|
|
|
char *do_select(SOCKET skt, bool startup)
|
|
{
|
|
int events;
|
|
if (startup) {
|
|
events = (FD_CONNECT | FD_READ | FD_WRITE |
|
|
FD_OOB | FD_CLOSE | FD_ACCEPT);
|
|
} else {
|
|
events = 0;
|
|
}
|
|
if (p_WSAEventSelect(skt, netevent, events) == SOCKET_ERROR) {
|
|
switch (p_WSAGetLastError()) {
|
|
case WSAENETDOWN:
|
|
return "Network is down";
|
|
default:
|
|
return "WSAEventSelect(): unknown error";
|
|
}
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
int stdin_gotdata(struct handle *h, void *data, int len)
|
|
{
|
|
if (len < 0) {
|
|
/*
|
|
* Special case: report read error.
|
|
*/
|
|
char buf[4096];
|
|
FormatMessage(FORMAT_MESSAGE_FROM_SYSTEM, NULL, -len, 0,
|
|
buf, lenof(buf), NULL);
|
|
buf[lenof(buf)-1] = '\0';
|
|
if (buf[strlen(buf)-1] == '\n')
|
|
buf[strlen(buf)-1] = '\0';
|
|
fprintf(stderr, "Unable to read from standard input: %s\n", buf);
|
|
cleanup_exit(0);
|
|
}
|
|
noise_ultralight(len);
|
|
if (backend_connected(backend)) {
|
|
if (len > 0) {
|
|
return backend_send(backend, data, len);
|
|
} else {
|
|
backend_special(backend, SS_EOF, 0);
|
|
return 0;
|
|
}
|
|
} else
|
|
return 0;
|
|
}
|
|
|
|
void stdouterr_sent(struct handle *h, int new_backlog)
|
|
{
|
|
if (new_backlog < 0) {
|
|
/*
|
|
* Special case: report write error.
|
|
*/
|
|
char buf[4096];
|
|
FormatMessage(FORMAT_MESSAGE_FROM_SYSTEM, NULL, -new_backlog, 0,
|
|
buf, lenof(buf), NULL);
|
|
buf[lenof(buf)-1] = '\0';
|
|
if (buf[strlen(buf)-1] == '\n')
|
|
buf[strlen(buf)-1] = '\0';
|
|
fprintf(stderr, "Unable to write to standard %s: %s\n",
|
|
(h == stdout_handle ? "output" : "error"), buf);
|
|
cleanup_exit(0);
|
|
}
|
|
if (backend_connected(backend)) {
|
|
backend_unthrottle(backend, (handle_backlog(stdout_handle) +
|
|
handle_backlog(stderr_handle)));
|
|
}
|
|
}
|
|
|
|
const bool share_can_be_downstream = true;
|
|
const bool share_can_be_upstream = true;
|
|
|
|
int main(int argc, char **argv)
|
|
{
|
|
bool sending;
|
|
SOCKET *sklist;
|
|
int skcount, sksize;
|
|
int exitcode;
|
|
bool errors;
|
|
bool use_subsystem = false;
|
|
bool just_test_share_exists = false;
|
|
unsigned long now, next, then;
|
|
const struct BackendVtable *vt;
|
|
|
|
dll_hijacking_protection();
|
|
|
|
sklist = NULL;
|
|
skcount = sksize = 0;
|
|
/*
|
|
* Initialise port and protocol to sensible defaults. (These
|
|
* will be overridden by more or less anything.)
|
|
*/
|
|
default_protocol = PROT_SSH;
|
|
default_port = 22;
|
|
|
|
flags = 0;
|
|
cmdline_tooltype |=
|
|
(TOOLTYPE_HOST_ARG |
|
|
TOOLTYPE_HOST_ARG_CAN_BE_SESSION |
|
|
TOOLTYPE_HOST_ARG_PROTOCOL_PREFIX |
|
|
TOOLTYPE_HOST_ARG_FROM_LAUNCHABLE_LOAD);
|
|
|
|
/*
|
|
* Process the command line.
|
|
*/
|
|
conf = conf_new();
|
|
do_defaults(NULL, conf);
|
|
loaded_session = false;
|
|
default_protocol = conf_get_int(conf, CONF_protocol);
|
|
default_port = conf_get_int(conf, CONF_port);
|
|
errors = false;
|
|
{
|
|
/*
|
|
* Override the default protocol if PLINK_PROTOCOL is set.
|
|
*/
|
|
char *p = getenv("PLINK_PROTOCOL");
|
|
if (p) {
|
|
const struct BackendVtable *vt = backend_vt_from_name(p);
|
|
if (vt) {
|
|
default_protocol = vt->protocol;
|
|
default_port = vt->default_port;
|
|
conf_set_int(conf, CONF_protocol, default_protocol);
|
|
conf_set_int(conf, CONF_port, default_port);
|
|
}
|
|
}
|
|
}
|
|
while (--argc) {
|
|
char *p = *++argv;
|
|
int ret = cmdline_process_param(p, (argc > 1 ? argv[1] : NULL),
|
|
1, conf);
|
|
if (ret == -2) {
|
|
fprintf(stderr,
|
|
"plink: option \"%s\" requires an argument\n", p);
|
|
errors = true;
|
|
} else if (ret == 2) {
|
|
--argc, ++argv;
|
|
} else if (ret == 1) {
|
|
continue;
|
|
} else if (!strcmp(p, "-batch")) {
|
|
console_batch_mode = true;
|
|
} else if (!strcmp(p, "-s")) {
|
|
/* Save status to write to conf later. */
|
|
use_subsystem = true;
|
|
} else if (!strcmp(p, "-V") || !strcmp(p, "--version")) {
|
|
version();
|
|
} else if (!strcmp(p, "--help")) {
|
|
usage();
|
|
} else if (!strcmp(p, "-pgpfp")) {
|
|
pgp_fingerprints();
|
|
exit(1);
|
|
} else if (!strcmp(p, "-shareexists")) {
|
|
just_test_share_exists = true;
|
|
} else if (*p != '-') {
|
|
char *command;
|
|
int cmdlen, cmdsize;
|
|
cmdlen = cmdsize = 0;
|
|
command = NULL;
|
|
|
|
while (argc) {
|
|
while (*p) {
|
|
if (cmdlen >= cmdsize) {
|
|
cmdsize = cmdlen + 512;
|
|
command = sresize(command, cmdsize, char);
|
|
}
|
|
command[cmdlen++]=*p++;
|
|
}
|
|
if (cmdlen >= cmdsize) {
|
|
cmdsize = cmdlen + 512;
|
|
command = sresize(command, cmdsize, char);
|
|
}
|
|
command[cmdlen++]=' '; /* always add trailing space */
|
|
if (--argc) p = *++argv;
|
|
}
|
|
if (cmdlen) command[--cmdlen]='\0';
|
|
/* change trailing blank to NUL */
|
|
conf_set_str(conf, CONF_remote_cmd, command);
|
|
conf_set_str(conf, CONF_remote_cmd2, "");
|
|
conf_set_bool(conf, CONF_nopty, true); /* command => no tty */
|
|
|
|
break; /* done with cmdline */
|
|
} else {
|
|
fprintf(stderr, "plink: unknown option \"%s\"\n", p);
|
|
errors = true;
|
|
}
|
|
}
|
|
|
|
if (errors)
|
|
return 1;
|
|
|
|
if (!cmdline_host_ok(conf)) {
|
|
usage();
|
|
}
|
|
|
|
prepare_session(conf);
|
|
|
|
/*
|
|
* Perform command-line overrides on session configuration.
|
|
*/
|
|
cmdline_run_saved(conf);
|
|
|
|
/*
|
|
* Apply subsystem status.
|
|
*/
|
|
if (use_subsystem)
|
|
conf_set_bool(conf, CONF_ssh_subsys, true);
|
|
|
|
if (!*conf_get_str(conf, CONF_remote_cmd) &&
|
|
!*conf_get_str(conf, CONF_remote_cmd2) &&
|
|
!*conf_get_str(conf, CONF_ssh_nc_host))
|
|
flags |= FLAG_INTERACTIVE;
|
|
|
|
/*
|
|
* Select protocol. This is farmed out into a table in a
|
|
* separate file to enable an ssh-free variant.
|
|
*/
|
|
vt = backend_vt_from_proto(conf_get_int(conf, CONF_protocol));
|
|
if (vt == NULL) {
|
|
fprintf(stderr,
|
|
"Internal fault: Unsupported protocol found\n");
|
|
return 1;
|
|
}
|
|
|
|
sk_init();
|
|
if (p_WSAEventSelect == NULL) {
|
|
fprintf(stderr, "Plink requires WinSock 2\n");
|
|
return 1;
|
|
}
|
|
|
|
/*
|
|
* Plink doesn't provide any way to add forwardings after the
|
|
* connection is set up, so if there are none now, we can safely set
|
|
* the "simple" flag.
|
|
*/
|
|
if (conf_get_int(conf, CONF_protocol) == PROT_SSH &&
|
|
!conf_get_bool(conf, CONF_x11_forward) &&
|
|
!conf_get_bool(conf, CONF_agentfwd) &&
|
|
!conf_get_str_nthstrkey(conf, CONF_portfwd, 0))
|
|
conf_set_bool(conf, CONF_ssh_simple, true);
|
|
|
|
logctx = log_init(default_logpolicy, conf);
|
|
|
|
if (just_test_share_exists) {
|
|
if (!vt->test_for_upstream) {
|
|
fprintf(stderr, "Connection sharing not supported for connection "
|
|
"type '%s'\n", vt->name);
|
|
return 1;
|
|
}
|
|
if (vt->test_for_upstream(conf_get_str(conf, CONF_host),
|
|
conf_get_int(conf, CONF_port), conf))
|
|
return 0;
|
|
else
|
|
return 1;
|
|
}
|
|
|
|
if (restricted_acl) {
|
|
lp_eventlog(default_logpolicy, "Running with restricted process ACL");
|
|
}
|
|
|
|
/*
|
|
* Start up the connection.
|
|
*/
|
|
netevent = CreateEvent(NULL, false, false, NULL);
|
|
{
|
|
const char *error;
|
|
char *realhost;
|
|
/* nodelay is only useful if stdin is a character device (console) */
|
|
bool nodelay = conf_get_bool(conf, CONF_tcp_nodelay) &&
|
|
(GetFileType(GetStdHandle(STD_INPUT_HANDLE)) == FILE_TYPE_CHAR);
|
|
|
|
error = backend_init(vt, plink_seat, &backend, logctx, conf,
|
|
conf_get_str(conf, CONF_host),
|
|
conf_get_int(conf, CONF_port),
|
|
&realhost, nodelay,
|
|
conf_get_bool(conf, CONF_tcp_keepalives));
|
|
if (error) {
|
|
fprintf(stderr, "Unable to open connection:\n%s", error);
|
|
return 1;
|
|
}
|
|
sfree(realhost);
|
|
}
|
|
|
|
inhandle = GetStdHandle(STD_INPUT_HANDLE);
|
|
outhandle = GetStdHandle(STD_OUTPUT_HANDLE);
|
|
errhandle = GetStdHandle(STD_ERROR_HANDLE);
|
|
|
|
/*
|
|
* Turn off ECHO and LINE input modes. We don't care if this
|
|
* call fails, because we know we aren't necessarily running in
|
|
* a console.
|
|
*/
|
|
GetConsoleMode(inhandle, &orig_console_mode);
|
|
SetConsoleMode(inhandle, ENABLE_PROCESSED_INPUT);
|
|
|
|
/*
|
|
* Pass the output handles to the handle-handling subsystem.
|
|
* (The input one we leave until we're through the
|
|
* authentication process.)
|
|
*/
|
|
stdout_handle = handle_output_new(outhandle, stdouterr_sent, NULL, 0);
|
|
stderr_handle = handle_output_new(errhandle, stdouterr_sent, NULL, 0);
|
|
|
|
main_thread_id = GetCurrentThreadId();
|
|
|
|
sending = false;
|
|
|
|
now = GETTICKCOUNT();
|
|
|
|
while (1) {
|
|
int nhandles;
|
|
HANDLE *handles;
|
|
int n;
|
|
DWORD ticks;
|
|
|
|
if (!sending && backend_sendok(backend)) {
|
|
stdin_handle = handle_input_new(inhandle, stdin_gotdata, NULL,
|
|
0);
|
|
sending = true;
|
|
}
|
|
|
|
if (toplevel_callback_pending()) {
|
|
ticks = 0;
|
|
next = now;
|
|
} else if (run_timers(now, &next)) {
|
|
then = now;
|
|
now = GETTICKCOUNT();
|
|
if (now - then > next - then)
|
|
ticks = 0;
|
|
else
|
|
ticks = next - now;
|
|
} else {
|
|
ticks = INFINITE;
|
|
/* no need to initialise next here because we can never
|
|
* get WAIT_TIMEOUT */
|
|
}
|
|
|
|
handles = handle_get_events(&nhandles);
|
|
handles = sresize(handles, nhandles+1, HANDLE);
|
|
handles[nhandles] = netevent;
|
|
n = MsgWaitForMultipleObjects(nhandles+1, handles, false, ticks,
|
|
QS_POSTMESSAGE);
|
|
if ((unsigned)(n - WAIT_OBJECT_0) < (unsigned)nhandles) {
|
|
handle_got_event(handles[n - WAIT_OBJECT_0]);
|
|
} else if (n == WAIT_OBJECT_0 + nhandles) {
|
|
WSANETWORKEVENTS things;
|
|
SOCKET socket;
|
|
extern SOCKET first_socket(int *), next_socket(int *);
|
|
int i, socketstate;
|
|
|
|
/*
|
|
* We must not call select_result() for any socket
|
|
* until we have finished enumerating within the tree.
|
|
* This is because select_result() may close the socket
|
|
* and modify the tree.
|
|
*/
|
|
/* Count the active sockets. */
|
|
i = 0;
|
|
for (socket = first_socket(&socketstate);
|
|
socket != INVALID_SOCKET;
|
|
socket = next_socket(&socketstate)) i++;
|
|
|
|
/* Expand the buffer if necessary. */
|
|
if (i > sksize) {
|
|
sksize = i + 16;
|
|
sklist = sresize(sklist, sksize, SOCKET);
|
|
}
|
|
|
|
/* Retrieve the sockets into sklist. */
|
|
skcount = 0;
|
|
for (socket = first_socket(&socketstate);
|
|
socket != INVALID_SOCKET;
|
|
socket = next_socket(&socketstate)) {
|
|
sklist[skcount++] = socket;
|
|
}
|
|
|
|
/* Now we're done enumerating; go through the list. */
|
|
for (i = 0; i < skcount; i++) {
|
|
WPARAM wp;
|
|
socket = sklist[i];
|
|
wp = (WPARAM) socket;
|
|
if (!p_WSAEnumNetworkEvents(socket, NULL, &things)) {
|
|
static const struct { int bit, mask; } eventtypes[] = {
|
|
{FD_CONNECT_BIT, FD_CONNECT},
|
|
{FD_READ_BIT, FD_READ},
|
|
{FD_CLOSE_BIT, FD_CLOSE},
|
|
{FD_OOB_BIT, FD_OOB},
|
|
{FD_WRITE_BIT, FD_WRITE},
|
|
{FD_ACCEPT_BIT, FD_ACCEPT},
|
|
};
|
|
int e;
|
|
|
|
noise_ultralight(socket);
|
|
noise_ultralight(things.lNetworkEvents);
|
|
|
|
for (e = 0; e < lenof(eventtypes); e++)
|
|
if (things.lNetworkEvents & eventtypes[e].mask) {
|
|
LPARAM lp;
|
|
int err = things.iErrorCode[eventtypes[e].bit];
|
|
lp = WSAMAKESELECTREPLY(eventtypes[e].mask, err);
|
|
select_result(wp, lp);
|
|
}
|
|
}
|
|
}
|
|
} else if (n == WAIT_OBJECT_0 + nhandles + 1) {
|
|
MSG msg;
|
|
while (PeekMessage(&msg, INVALID_HANDLE_VALUE,
|
|
WM_AGENT_CALLBACK, WM_AGENT_CALLBACK,
|
|
PM_REMOVE)) {
|
|
struct agent_callback *c = (struct agent_callback *)msg.lParam;
|
|
c->callback(c->callback_ctx, c->data, c->len);
|
|
sfree(c);
|
|
}
|
|
}
|
|
|
|
run_toplevel_callbacks();
|
|
|
|
if (n == WAIT_TIMEOUT) {
|
|
now = next;
|
|
} else {
|
|
now = GETTICKCOUNT();
|
|
}
|
|
|
|
sfree(handles);
|
|
|
|
if (sending)
|
|
handle_unthrottle(stdin_handle, backend_sendbuffer(backend));
|
|
|
|
if (!backend_connected(backend) &&
|
|
handle_backlog(stdout_handle) + handle_backlog(stderr_handle) == 0)
|
|
break; /* we closed the connection */
|
|
}
|
|
exitcode = backend_exitcode(backend);
|
|
if (exitcode < 0) {
|
|
fprintf(stderr, "Remote process exit code unavailable\n");
|
|
exitcode = 1; /* this is an error condition */
|
|
}
|
|
cleanup_exit(exitcode);
|
|
return 0; /* placate compiler warning */
|
|
}
|