mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-11 16:23:55 -05:00
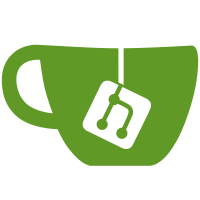
message_box() previously differed from the real MessageBox API function in that it permitted the user to provide a help context to be used for a Help button in the dialog box. Now it adds a second unusual ability: you can specify that the text and caption strings are in UTF-8 rather than the system code page.
72 lines
2.1 KiB
C
72 lines
2.1 KiB
C
/*
|
|
* Enhanced version of the MessageBox API function. Permits enabling a
|
|
* Help button by setting helpctxid to a context id in the help file
|
|
* relevant to this dialog box. Also permits setting the 'utf8' flag
|
|
* to indicate that the char strings given as 'text' and 'caption' are
|
|
* encoded in UTF-8 rather than the system code page.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
static HWND message_box_owner;
|
|
|
|
/* Callback function to launch context help. */
|
|
static VOID CALLBACK message_box_help_callback(LPHELPINFO lpHelpInfo)
|
|
{
|
|
const char *context = NULL;
|
|
#define CHECK_CTX(name) \
|
|
do { \
|
|
if (lpHelpInfo->dwContextId == WINHELP_CTXID_ ## name) \
|
|
context = WINHELP_CTX_ ## name; \
|
|
} while (0)
|
|
CHECK_CTX(errors_hostkey_absent);
|
|
CHECK_CTX(errors_hostkey_changed);
|
|
CHECK_CTX(errors_cantloadkey);
|
|
CHECK_CTX(option_cleanup);
|
|
CHECK_CTX(pgp_fingerprints);
|
|
#undef CHECK_CTX
|
|
if (context)
|
|
launch_help(message_box_owner, context);
|
|
}
|
|
|
|
int message_box(HWND owner, LPCTSTR text, LPCTSTR caption, DWORD style,
|
|
bool utf8, DWORD helpctxid)
|
|
{
|
|
MSGBOXPARAMSW mbox;
|
|
|
|
/*
|
|
* We use MessageBoxIndirect() because it allows us to specify a
|
|
* callback function for the Help button.
|
|
*/
|
|
mbox.cbSize = sizeof(mbox);
|
|
/* Assumes the globals `hinst' and `hwnd' have sensible values. */
|
|
mbox.hInstance = hinst;
|
|
mbox.dwLanguageId = LANG_NEUTRAL;
|
|
|
|
mbox.hwndOwner = message_box_owner = owner;
|
|
|
|
wchar_t *wtext, *wcaption;
|
|
if (utf8) {
|
|
wtext = decode_utf8_to_wide_string(text);
|
|
wcaption = decode_utf8_to_wide_string(caption);
|
|
} else {
|
|
wtext = dup_mb_to_wc(DEFAULT_CODEPAGE, 0, text);
|
|
wcaption = dup_mb_to_wc(DEFAULT_CODEPAGE, 0, caption);
|
|
}
|
|
mbox.lpszText = wtext;
|
|
mbox.lpszCaption = wcaption;
|
|
|
|
mbox.dwStyle = style;
|
|
|
|
mbox.dwContextHelpId = helpctxid;
|
|
if (helpctxid != 0 && has_help()) mbox.dwStyle |= MB_HELP;
|
|
mbox.lpfnMsgBoxCallback = &message_box_help_callback;
|
|
|
|
int toret = MessageBoxIndirectW(&mbox);
|
|
|
|
sfree(wtext);
|
|
sfree(wcaption);
|
|
|
|
return toret;
|
|
}
|