mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
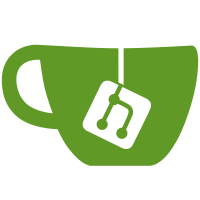
This is a sweeping change applied across the whole code base by a spot of Emacs Lisp. Now, everywhere I declare a vtable filled with function pointers (and the occasional const data member), all the members of the vtable structure are initialised by name using the '.fieldname = value' syntax introduced in C99. We were already using this syntax for a handful of things in the new key-generation progress report system, so it's not new to the code base as a whole. The advantage is that now, when a vtable only declares a subset of the available fields, I can initialise the rest to NULL or zero just by leaving them out. This is most dramatic in a couple of the outlying vtables in things like psocks (which has a ConnectionLayerVtable containing only one non-NULL method), but less dramatically, it means that the new 'flags' field in BackendVtable can be completely left out of every backend definition except for the SUPDUP one which defines it to a nonzero value. Similarly, the test_for_upstream method only used by SSH doesn't have to be mentioned in the rest of the backends; network Plugs for listening sockets don't have to explicitly null out 'receive' and 'sent', and vice versa for 'accepting', and so on. While I'm at it, I've normalised the declarations so they don't use the unnecessarily verbose 'struct' keyword. Also a handful of them weren't const; now they are.
75 lines
1.4 KiB
C
75 lines
1.4 KiB
C
/*
|
|
* A dummy Socket implementation which just holds an error message.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <assert.h>
|
|
|
|
#include "tree234.h"
|
|
#include "putty.h"
|
|
#include "network.h"
|
|
|
|
typedef struct {
|
|
char *error;
|
|
Plug *plug;
|
|
|
|
Socket sock;
|
|
} ErrorSocket;
|
|
|
|
static Plug *sk_error_plug(Socket *s, Plug *p)
|
|
{
|
|
ErrorSocket *es = container_of(s, ErrorSocket, sock);
|
|
Plug *ret = es->plug;
|
|
if (p)
|
|
es->plug = p;
|
|
return ret;
|
|
}
|
|
|
|
static void sk_error_close(Socket *s)
|
|
{
|
|
ErrorSocket *es = container_of(s, ErrorSocket, sock);
|
|
|
|
sfree(es->error);
|
|
sfree(es);
|
|
}
|
|
|
|
static const char *sk_error_socket_error(Socket *s)
|
|
{
|
|
ErrorSocket *es = container_of(s, ErrorSocket, sock);
|
|
return es->error;
|
|
}
|
|
|
|
static SocketPeerInfo *sk_error_peer_info(Socket *s)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static const SocketVtable ErrorSocket_sockvt = {
|
|
.plug = sk_error_plug,
|
|
.close = sk_error_close,
|
|
.socket_error = sk_error_socket_error,
|
|
.peer_info = sk_error_peer_info,
|
|
/* other methods are NULL */
|
|
};
|
|
|
|
Socket *new_error_socket_consume_string(Plug *plug, char *errmsg)
|
|
{
|
|
ErrorSocket *es = snew(ErrorSocket);
|
|
es->sock.vt = &ErrorSocket_sockvt;
|
|
es->plug = plug;
|
|
es->error = errmsg;
|
|
return &es->sock;
|
|
}
|
|
|
|
Socket *new_error_socket_fmt(Plug *plug, const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
char *msg;
|
|
|
|
va_start(ap, fmt);
|
|
msg = dupvprintf(fmt, ap);
|
|
va_end(ap);
|
|
|
|
return new_error_socket_consume_string(plug, msg);
|
|
}
|