mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
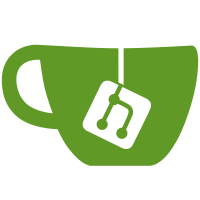
The old API was one of those horrible things I used to do when I was
young and foolish, in which you have just one function, and indicate
which of lots of things it's doing by passing in flags. It was crying
out to be replaced with a vtable.
While I'm at it, I've reworked the code on the Windows side that
decides what to do with the progress bar, so that it's based on
actually justifiable estimates of probability rather than magic
integer constants.
Since computers are generally faster now than they were at the start
of this project, I've also decided there's no longer any point in
making the fixed final part of RSA key generation bother to report
progress at all. So the progress bars are now only for the variable
part, i.e. the actual prime generations.
(This is a reapplication of commit a7bdefb39
, without the Miller-Rabin
refactoring accidentally folded into it. Also this time I've added -lm
to the link options, which for some reason _didn't_ cause me a link
failure last time round. No idea why not.)
38 lines
916 B
C
38 lines
916 B
C
/*
|
|
* EC key generation.
|
|
*/
|
|
|
|
#include "ssh.h"
|
|
#include "sshkeygen.h"
|
|
#include "mpint.h"
|
|
|
|
int ecdsa_generate(struct ecdsa_key *ek, int bits)
|
|
{
|
|
if (!ec_nist_alg_and_curve_by_bits(bits, &ek->curve, &ek->sshk.vt))
|
|
return 0;
|
|
|
|
mp_int *one = mp_from_integer(1);
|
|
ek->privateKey = mp_random_in_range(one, ek->curve->w.G_order);
|
|
mp_free(one);
|
|
|
|
ek->publicKey = ecdsa_public(ek->privateKey, ek->sshk.vt);
|
|
|
|
return 1;
|
|
}
|
|
|
|
int eddsa_generate(struct eddsa_key *ek, int bits)
|
|
{
|
|
if (!ec_ed_alg_and_curve_by_bits(bits, &ek->curve, &ek->sshk.vt))
|
|
return 0;
|
|
|
|
/* EdDSA secret keys are just 32 bytes of hash preimage; the
|
|
* 64-byte SHA-512 hash of that key will be used when signing,
|
|
* but the form of the key stored on disk is the preimage
|
|
* only. */
|
|
ek->privateKey = mp_random_bits(bits);
|
|
|
|
ek->publicKey = eddsa_public(ek->privateKey, ek->sshk.vt);
|
|
|
|
return 1;
|
|
}
|