mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-11 08:13:46 -05:00
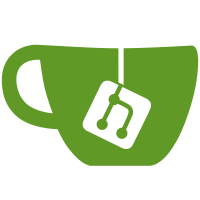
I've defined a new value for the 'int type' parameter passed to plug_log(), which proxy sockets will use to pass their backend information on how the setup of their proxied connections are going. I've implemented support for the new type code in all _nontrivial_ plug log functions (which, conveniently, are precisely the ones I just refactored into backend_socket_log); the ones which just throw all their log data away anyway will do that to the new code as well. We use the new type code to log the DNS lookup and connection setup for connecting to a networked proxy, and also to log the exact command string sent down Telnet proxy connections (so the user can easily debug mistakes in the configured format string) and the exact command executed when spawning a local proxy process. (The latter was already supported on Windows by a bodgy logging call taking advantage of Windows in particular having no front end pointer; I've converted that into a sensible use of the new plug_log facility, and done the same thing on Unix.)
42 lines
1.1 KiB
C
42 lines
1.1 KiB
C
/*
|
|
* be_misc.c: helper functions shared between main network backends.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
#include "network.h"
|
|
|
|
void backend_socket_log(void *frontend, int type, SockAddr addr, int port,
|
|
const char *error_msg, int error_code)
|
|
{
|
|
char addrbuf[256], *msg;
|
|
|
|
switch (type) {
|
|
case 0:
|
|
sk_getaddr(addr, addrbuf, lenof(addrbuf));
|
|
if (sk_addr_needs_port(addr)) {
|
|
msg = dupprintf("Connecting to %s port %d", addrbuf, port);
|
|
} else {
|
|
msg = dupprintf("Connecting to %s", addrbuf);
|
|
}
|
|
break;
|
|
case 1:
|
|
sk_getaddr(addr, addrbuf, lenof(addrbuf));
|
|
msg = dupprintf("Failed to connect to %s: %s", addrbuf, error_msg);
|
|
break;
|
|
case 2:
|
|
/* Proxy-related log messages have their own identifying
|
|
* prefix already, put on by our caller. */
|
|
msg = dupstr(error_msg);
|
|
break;
|
|
default:
|
|
msg = NULL; /* shouldn't happen, but placate optimiser */
|
|
break;
|
|
}
|
|
|
|
if (msg) {
|
|
logevent(frontend, msg);
|
|
sfree(msg);
|
|
}
|
|
}
|
|
|