mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-05 13:32:48 -05:00
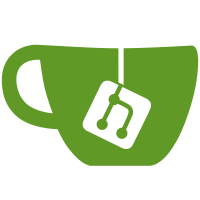
Now, when you resize the Event Log window, the list box grows in both directions. Previously, as a side effect of the Columns-based layout, it grew only horizontally. I've arranged this by adding an extra wrinkle to the Columns layout system, which allows you to tag _exactly one_ widget in the whole container as the 'vexpand' widget. When the Columns is size-allocated taller than its minimum height, the vexpand widget is given all the extra space. This technique ports naturally across all versions of GTK (since the hard part is done in my own code). But it's limited: you can't set more than one widget to be vexpand (which saves having to figure out whether they're side by side and can expand in parallel, or vertically separated and each have to get half the available extra space, etc). And in complex layouts where the widget you really want to expand is in a sub-Columns, there's no system for recursively searching down to find it. In other words, this is a one-shot bodge for the Event Log, and it will want more work if we ever plan to extend it to list boxes in the main config dialog.
68 lines
2.2 KiB
C
68 lines
2.2 KiB
C
/*
|
|
* columns.h - header file for a columns-based widget container
|
|
* capable of supporting the PuTTY portable dialog box layout
|
|
* mechanism.
|
|
*/
|
|
|
|
#ifndef COLUMNS_H
|
|
#define COLUMNS_H
|
|
|
|
#include <gdk/gdk.h>
|
|
#include <gtk/gtk.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif /* __cplusplus */
|
|
|
|
#define TYPE_COLUMNS (columns_get_type())
|
|
#define COLUMNS(obj) (G_TYPE_CHECK_INSTANCE_CAST((obj), TYPE_COLUMNS, Columns))
|
|
#define COLUMNS_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_CAST((klass), TYPE_COLUMNS, ColumnsClass))
|
|
#define IS_COLUMNS(obj) (G_TYPE_CHECK_INSTANCE_TYPE((obj), TYPE_COLUMNS))
|
|
#define IS_COLUMNS_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE((klass), TYPE_COLUMNS))
|
|
|
|
typedef struct Columns_tag Columns;
|
|
typedef struct ColumnsClass_tag ColumnsClass;
|
|
typedef struct ColumnsChild_tag ColumnsChild;
|
|
|
|
struct Columns_tag {
|
|
GtkContainer container;
|
|
/* private after here */
|
|
GList *children; /* this holds ColumnsChild structures */
|
|
GList *taborder; /* this just holds GtkWidgets */
|
|
ColumnsChild *vexpand;
|
|
gint spacing;
|
|
};
|
|
|
|
struct ColumnsClass_tag {
|
|
GtkContainerClass parent_class;
|
|
};
|
|
|
|
struct ColumnsChild_tag {
|
|
/* If `widget' is non-NULL, this entry represents an actual widget. */
|
|
GtkWidget *widget;
|
|
gint colstart, colspan;
|
|
bool force_left; /* for recalcitrant GtkLabels */
|
|
ColumnsChild *same_height_as;
|
|
/* Otherwise, this entry represents a change in the column setup. */
|
|
gint ncols;
|
|
gint *percentages;
|
|
gint x, y, w, h; /* used during an individual size computation */
|
|
};
|
|
|
|
GType columns_get_type(void);
|
|
GtkWidget *columns_new(gint spacing);
|
|
void columns_set_cols(Columns *cols, gint ncols, const gint *percentages);
|
|
void columns_add(Columns *cols, GtkWidget *child,
|
|
gint colstart, gint colspan);
|
|
void columns_taborder_last(Columns *cols, GtkWidget *child);
|
|
void columns_force_left_align(Columns *cols, GtkWidget *child);
|
|
void columns_force_same_height(Columns *cols, GtkWidget *ch1, GtkWidget *ch2);
|
|
void columns_vexpand(Columns *cols, GtkWidget *child);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif /* __cplusplus */
|
|
|
|
#endif /* COLUMNS_H */
|