mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-15 18:17:32 -05:00
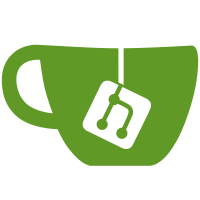
After Pavel Kryukov pointed out that I have to put _something_ in the 'ssh_key' structure, I thought of an actually useful thing to put there: why not make it store a pointer to the ssh_keyalg structure? Then ssh_key becomes a classoid - or perhaps 'traitoid' is a closer analogy - in the same style as Socket and Plug. And just like Socket and Plug, I've also arranged a system of wrapper macros that avoid the need to mention the 'object' whose method you're invoking twice at each call site. The new vtable pointer directly replaces an existing field of struct ec_key (which was usable by several different ssh_keyalgs, so it already had to store a pointer to the currently active one), and also replaces the 'alg' field of the ssh2_userkey structure that wraps up a cryptographic key with its comment field. I've also taken the opportunity to clean things up a bit in general: most of the methods now have new and clearer names (e.g. you'd never know that 'newkey' made a public-only key while 'createkey' made a public+private key pair unless you went and looked it up, but now they're called 'new_pub' and 'new_priv' you might be in with a chance), and I've completely removed the openssh_private_npieces field after realising that it was duplicating information that is actually _more_ conveniently obtained by calling the new_priv_openssh method (formerly openssh_createkey) and throwing away the result.
72 lines
1.8 KiB
C
72 lines
1.8 KiB
C
/*
|
|
* EC key generation.
|
|
*/
|
|
|
|
#include "ssh.h"
|
|
|
|
/* Forward reference from sshecc.c */
|
|
struct ec_point *ecp_mul(const struct ec_point *a, const Bignum b);
|
|
|
|
int ec_generate(struct ec_key *key, int bits, progfn_t pfn,
|
|
void *pfnparam)
|
|
{
|
|
struct ec_point *publicKey;
|
|
|
|
if (!ec_nist_alg_and_curve_by_bits(bits, &key->publicKey.curve,
|
|
&key->sshk))
|
|
return 0;
|
|
|
|
key->privateKey = bignum_random_in_range(One, key->publicKey.curve->w.n);
|
|
if (!key->privateKey) return 0;
|
|
|
|
publicKey = ec_public(key->privateKey, key->publicKey.curve);
|
|
if (!publicKey) {
|
|
freebn(key->privateKey);
|
|
key->privateKey = NULL;
|
|
return 0;
|
|
}
|
|
|
|
key->publicKey.x = publicKey->x;
|
|
key->publicKey.y = publicKey->y;
|
|
key->publicKey.z = NULL;
|
|
sfree(publicKey);
|
|
|
|
return 1;
|
|
}
|
|
|
|
int ec_edgenerate(struct ec_key *key, int bits, progfn_t pfn,
|
|
void *pfnparam)
|
|
{
|
|
struct ec_point *publicKey;
|
|
|
|
if (!ec_ed_alg_and_curve_by_bits(bits, &key->publicKey.curve,
|
|
&key->sshk))
|
|
return 0;
|
|
|
|
{
|
|
/* EdDSA secret keys are just 32 bytes of hash preimage; the
|
|
* 64-byte SHA-512 hash of that key will be used when signing,
|
|
* but the form of the key stored on disk is the preimage
|
|
* only. */
|
|
Bignum privMax = bn_power_2(bits);
|
|
if (!privMax) return 0;
|
|
key->privateKey = bignum_random_in_range(Zero, privMax);
|
|
freebn(privMax);
|
|
if (!key->privateKey) return 0;
|
|
}
|
|
|
|
publicKey = ec_public(key->privateKey, key->publicKey.curve);
|
|
if (!publicKey) {
|
|
freebn(key->privateKey);
|
|
key->privateKey = NULL;
|
|
return 0;
|
|
}
|
|
|
|
key->publicKey.x = publicKey->x;
|
|
key->publicKey.y = publicKey->y;
|
|
key->publicKey.z = NULL;
|
|
sfree(publicKey);
|
|
|
|
return 1;
|
|
}
|