mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
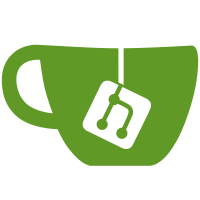
Some upcoming restructuring I've got planned will need to pass output packets back and forth on queues, as well as input ones. So here's a change that arranges that we can have a PktInQueue and a PktOutQueue, sharing most of their implementation via a PacketQueueBase structure which links together the PacketQueueNode fields in the two packet structures. There's a tricksy bit of macro manoeuvring to get all of this type-checked, so that I can't accidentally link a PktOut on to a PktInQueue or vice versa. It works by having the main queue functions wrapped by macros; when receiving a packet structure on input, they type-check it against the queue structure and then automatically look up its qnode field to pass to the underlying PacketQueueBase function; on output, they translate a returned PacketQueueNode back to its containing packet type by calling a 'get' function pointer.
71 lines
2.5 KiB
C
71 lines
2.5 KiB
C
/*
|
|
* Abstraction of the binary packet protocols used in SSH.
|
|
*/
|
|
|
|
#ifndef PUTTY_SSHBPP_H
|
|
#define PUTTY_SSHBPP_H
|
|
|
|
typedef struct BinaryPacketProtocol BinaryPacketProtocol;
|
|
|
|
struct BinaryPacketProtocolVtable {
|
|
void (*free)(BinaryPacketProtocol *);
|
|
void (*handle_input)(BinaryPacketProtocol *);
|
|
PktOut *(*new_pktout)(int type);
|
|
void (*format_packet)(BinaryPacketProtocol *, PktOut *);
|
|
};
|
|
|
|
struct BinaryPacketProtocol {
|
|
const struct BinaryPacketProtocolVtable *vt;
|
|
bufchain *in_raw, *out_raw;
|
|
PktInQueue *in_pq;
|
|
PacketLogSettings *pls;
|
|
LogContext *logctx;
|
|
|
|
int seen_disconnect;
|
|
char *error;
|
|
};
|
|
|
|
#define ssh_bpp_free(bpp) ((bpp)->vt->free(bpp))
|
|
#define ssh_bpp_handle_input(bpp) ((bpp)->vt->handle_input(bpp))
|
|
#define ssh_bpp_new_pktout(bpp, type) ((bpp)->vt->new_pktout(type))
|
|
#define ssh_bpp_format_packet(bpp, pkt) ((bpp)->vt->format_packet(bpp, pkt))
|
|
|
|
BinaryPacketProtocol *ssh1_bpp_new(void);
|
|
void ssh1_bpp_new_cipher(BinaryPacketProtocol *bpp,
|
|
const struct ssh1_cipheralg *cipher,
|
|
const void *session_key);
|
|
void ssh1_bpp_start_compression(BinaryPacketProtocol *bpp);
|
|
|
|
BinaryPacketProtocol *ssh2_bpp_new(void);
|
|
void ssh2_bpp_new_outgoing_crypto(
|
|
BinaryPacketProtocol *bpp,
|
|
const struct ssh2_cipheralg *cipher, const void *ckey, const void *iv,
|
|
const struct ssh2_macalg *mac, int etm_mode, const void *mac_key,
|
|
const struct ssh_compression_alg *compression);
|
|
void ssh2_bpp_new_incoming_crypto(
|
|
BinaryPacketProtocol *bpp,
|
|
const struct ssh2_cipheralg *cipher, const void *ckey, const void *iv,
|
|
const struct ssh2_macalg *mac, int etm_mode, const void *mac_key,
|
|
const struct ssh_compression_alg *compression);
|
|
|
|
BinaryPacketProtocol *ssh2_bare_bpp_new(void);
|
|
|
|
/*
|
|
* The initial code to handle the SSH version exchange is also
|
|
* structured as an implementation of BinaryPacketProtocol, because
|
|
* that makes it easy to switch from that to the next BPP once it
|
|
* tells us which one we're using.
|
|
*/
|
|
struct ssh_version_receiver {
|
|
void (*got_ssh_version)(struct ssh_version_receiver *rcv,
|
|
int major_version);
|
|
};
|
|
BinaryPacketProtocol *ssh_verstring_new(
|
|
Conf *conf, Frontend *frontend, int bare_connection_mode,
|
|
const char *protoversion, struct ssh_version_receiver *rcv);
|
|
const char *ssh_verstring_get_remote(BinaryPacketProtocol *);
|
|
const char *ssh_verstring_get_local(BinaryPacketProtocol *);
|
|
int ssh_verstring_get_bugs(BinaryPacketProtocol *);
|
|
|
|
#endif /* PUTTY_SSHBPP_H */
|