mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-09 23:33:46 -05:00
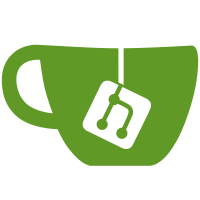
Now that all the call sites are expecting a size_t instead of an int length field, it's no longer particularly difficult to make it actually return the pointer,length pair in the form of a ptrlen. It would be nice to say that simplifies call sites because those ptrlens can all be passed straight along to other ptrlen-consuming functions. Actually almost none of the call sites are like that _yet_, but this makes it possible to move them in that direction in future (as part of my general aim to migrate ptrlen-wards as much as I can). But also it's just nicer to keep the pointer and length together in one variable, and not have to declare them both in advance with two extra lines of boilerplate.
39 lines
625 B
C
39 lines
625 B
C
/*
|
|
* Stub implementation of the printing interface for PuTTY, for the
|
|
* benefit of non-printing terminal applications.
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <stdio.h>
|
|
#include "putty.h"
|
|
|
|
struct printer_job_tag {
|
|
int dummy;
|
|
};
|
|
|
|
printer_job *printer_start_job(char *printer)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
void printer_job_data(printer_job *pj, void *data, size_t len)
|
|
{
|
|
}
|
|
|
|
void printer_finish_job(printer_job *pj)
|
|
{
|
|
}
|
|
|
|
printer_enum *printer_start_enum(int *nprinters_ptr)
|
|
{
|
|
*nprinters_ptr = 0;
|
|
return NULL;
|
|
}
|
|
char *printer_get_name(printer_enum *pe, int i)
|
|
{
|
|
return NULL;
|
|
}
|
|
void printer_finish_enum(printer_enum *pe)
|
|
{
|
|
}
|