mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
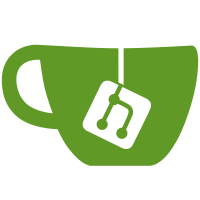
LogContext is now the owner of the logevent() function that back ends and so forth are constantly calling. Previously, logevent was owned by the Frontend, which would store the message into its list for the GUI Event Log dialog (or print it to standard error, or whatever) and then pass it _back_ to LogContext to write to the currently open log file. Now it's the other way round: LogContext gets the message from the back end first, writes it to its log file if it feels so inclined, and communicates it back to the front end. This means that lots of parts of the back end system no longer need to have a pointer to a full-on Frontend; the only thing they needed it for was logging, so now they just have a LogContext (which many of them had to have anyway, e.g. for logging SSH packets or session traffic). LogContext itself also doesn't get a full Frontend pointer any more: it now talks back to the front end via a little vtable of its own called LogPolicy, which contains the method that passes Event Log entries through, the old askappend() function that decides whether to truncate a pre-existing log file, and an emergency function for printing an especially prominent message if the log file can't be created. One minor nice effect of this is that console and GUI apps can implement that last function subtly differently, so that Unix console apps can write it with a plain \n instead of the \r\n (harmless but inelegant) that the old centralised implementation generated. One other consequence of this is that the LogContext has to be provided to backend_init() so that it's available to backends from the instant of creation, rather than being provided via a separate API call a couple of function calls later, because backends have typically started doing things that need logging (like making network connections) before the call to backend_provide_logctx. Fortunately, there's no case in the whole code base where we don't already have logctx by the time we make a backend (so I don't actually remember why I ever delayed providing one). So that shortens the backend API by one function, which is always nice. While I'm tidying up, I've also moved the printf-style logeventf() and the handy logevent_and_free() into logging.c, instead of having copies of them scattered around other places. This has also let me remove some stub functions from a couple of outlying applications like Pageant. Finally, I've removed the pointless "_tag" at the end of LogContext's official struct name.
187 lines
5.7 KiB
C
187 lines
5.7 KiB
C
/*
|
|
* Trivial binary packet protocol for the 'bare' ssh-connection
|
|
* protocol used in PuTTY's SSH-2 connection sharing system.
|
|
*/
|
|
|
|
#include <assert.h>
|
|
|
|
#include "putty.h"
|
|
#include "ssh.h"
|
|
#include "sshbpp.h"
|
|
#include "sshcr.h"
|
|
|
|
struct ssh2_bare_bpp_state {
|
|
int crState;
|
|
long packetlen, maxlen;
|
|
unsigned char *data;
|
|
unsigned long incoming_sequence, outgoing_sequence;
|
|
PktIn *pktin;
|
|
|
|
BinaryPacketProtocol bpp;
|
|
};
|
|
|
|
static void ssh2_bare_bpp_free(BinaryPacketProtocol *bpp);
|
|
static void ssh2_bare_bpp_handle_input(BinaryPacketProtocol *bpp);
|
|
static void ssh2_bare_bpp_handle_output(BinaryPacketProtocol *bpp);
|
|
static PktOut *ssh2_bare_bpp_new_pktout(int type);
|
|
|
|
static const struct BinaryPacketProtocolVtable ssh2_bare_bpp_vtable = {
|
|
ssh2_bare_bpp_free,
|
|
ssh2_bare_bpp_handle_input,
|
|
ssh2_bare_bpp_handle_output,
|
|
ssh2_bare_bpp_new_pktout,
|
|
ssh2_bpp_queue_disconnect, /* in sshcommon.c */
|
|
};
|
|
|
|
BinaryPacketProtocol *ssh2_bare_bpp_new(LogContext *logctx)
|
|
{
|
|
struct ssh2_bare_bpp_state *s = snew(struct ssh2_bare_bpp_state);
|
|
memset(s, 0, sizeof(*s));
|
|
s->bpp.vt = &ssh2_bare_bpp_vtable;
|
|
s->bpp.logctx = logctx;
|
|
ssh_bpp_common_setup(&s->bpp);
|
|
return &s->bpp;
|
|
}
|
|
|
|
static void ssh2_bare_bpp_free(BinaryPacketProtocol *bpp)
|
|
{
|
|
struct ssh2_bare_bpp_state *s =
|
|
container_of(bpp, struct ssh2_bare_bpp_state, bpp);
|
|
sfree(s->pktin);
|
|
sfree(s);
|
|
}
|
|
|
|
#define BPP_READ(ptr, len) do \
|
|
{ \
|
|
crMaybeWaitUntilV(s->bpp.input_eof || \
|
|
bufchain_try_fetch_consume( \
|
|
s->bpp.in_raw, ptr, len)); \
|
|
if (s->bpp.input_eof) \
|
|
goto eof; \
|
|
} while (0)
|
|
|
|
static void ssh2_bare_bpp_handle_input(BinaryPacketProtocol *bpp)
|
|
{
|
|
struct ssh2_bare_bpp_state *s =
|
|
container_of(bpp, struct ssh2_bare_bpp_state, bpp);
|
|
|
|
crBegin(s->crState);
|
|
|
|
while (1) {
|
|
/* Read the length field. */
|
|
{
|
|
unsigned char lenbuf[4];
|
|
BPP_READ(lenbuf, 4);
|
|
s->packetlen = toint(GET_32BIT_MSB_FIRST(lenbuf));
|
|
}
|
|
|
|
if (s->packetlen <= 0 || s->packetlen >= (long)OUR_V2_PACKETLIMIT) {
|
|
ssh_sw_abort(s->bpp.ssh, "Invalid packet length received");
|
|
crStopV;
|
|
}
|
|
|
|
/*
|
|
* Allocate the packet to return, now we know its length.
|
|
*/
|
|
s->pktin = snew_plus(PktIn, s->packetlen);
|
|
s->pktin->qnode.prev = s->pktin->qnode.next = NULL;
|
|
s->pktin->qnode.on_free_queue = FALSE;
|
|
s->maxlen = 0;
|
|
s->data = snew_plus_get_aux(s->pktin);
|
|
|
|
s->pktin->sequence = s->incoming_sequence++;
|
|
|
|
/*
|
|
* Read the remainder of the packet.
|
|
*/
|
|
BPP_READ(s->data, s->packetlen);
|
|
|
|
/*
|
|
* The data we just read is precisely the initial type byte
|
|
* followed by the packet payload.
|
|
*/
|
|
s->pktin->type = s->data[0];
|
|
s->data++;
|
|
s->packetlen--;
|
|
BinarySource_INIT(s->pktin, s->data, s->packetlen);
|
|
|
|
/*
|
|
* Log incoming packet, possibly omitting sensitive fields.
|
|
*/
|
|
if (s->bpp.logctx) {
|
|
logblank_t blanks[MAX_BLANKS];
|
|
int nblanks = ssh2_censor_packet(
|
|
s->bpp.pls, s->pktin->type, FALSE,
|
|
make_ptrlen(s->data, s->packetlen), blanks);
|
|
log_packet(s->bpp.logctx, PKT_INCOMING, s->pktin->type,
|
|
ssh2_pkt_type(s->bpp.pls->kctx, s->bpp.pls->actx,
|
|
s->pktin->type),
|
|
get_ptr(s->pktin), get_avail(s->pktin), nblanks, blanks,
|
|
&s->pktin->sequence, 0, NULL);
|
|
}
|
|
|
|
if (ssh2_bpp_check_unimplemented(&s->bpp, s->pktin)) {
|
|
sfree(s->pktin);
|
|
s->pktin = NULL;
|
|
continue;
|
|
}
|
|
|
|
pq_push(&s->bpp.in_pq, s->pktin);
|
|
s->pktin = NULL;
|
|
}
|
|
|
|
eof:
|
|
if (!s->bpp.expect_close) {
|
|
ssh_remote_error(s->bpp.ssh,
|
|
"Server unexpectedly closed network connection");
|
|
} else {
|
|
ssh_remote_eof(s->bpp.ssh, "Server closed network connection");
|
|
}
|
|
return; /* avoid touching s now it's been freed */
|
|
|
|
crFinishV;
|
|
}
|
|
|
|
static PktOut *ssh2_bare_bpp_new_pktout(int pkt_type)
|
|
{
|
|
PktOut *pkt = ssh_new_packet();
|
|
pkt->length = 4; /* space for packet length */
|
|
pkt->type = pkt_type;
|
|
put_byte(pkt, pkt_type);
|
|
return pkt;
|
|
}
|
|
|
|
static void ssh2_bare_bpp_format_packet(struct ssh2_bare_bpp_state *s,
|
|
PktOut *pkt)
|
|
{
|
|
if (s->bpp.logctx) {
|
|
ptrlen pktdata = make_ptrlen(pkt->data + 5, pkt->length - 5);
|
|
logblank_t blanks[MAX_BLANKS];
|
|
int nblanks = ssh2_censor_packet(
|
|
s->bpp.pls, pkt->type, TRUE, pktdata, blanks);
|
|
log_packet(s->bpp.logctx, PKT_OUTGOING, pkt->type,
|
|
ssh2_pkt_type(s->bpp.pls->kctx, s->bpp.pls->actx,
|
|
pkt->type),
|
|
pktdata.ptr, pktdata.len, nblanks, blanks,
|
|
&s->outgoing_sequence,
|
|
pkt->downstream_id, pkt->additional_log_text);
|
|
}
|
|
|
|
s->outgoing_sequence++; /* only for diagnostics, really */
|
|
|
|
PUT_32BIT(pkt->data, pkt->length - 4);
|
|
bufchain_add(s->bpp.out_raw, pkt->data, pkt->length);
|
|
}
|
|
|
|
static void ssh2_bare_bpp_handle_output(BinaryPacketProtocol *bpp)
|
|
{
|
|
struct ssh2_bare_bpp_state *s =
|
|
container_of(bpp, struct ssh2_bare_bpp_state, bpp);
|
|
PktOut *pkt;
|
|
|
|
while ((pkt = pq_pop(&s->bpp.out_pq)) != NULL) {
|
|
ssh2_bare_bpp_format_packet(s, pkt);
|
|
ssh_free_pktout(pkt);
|
|
}
|
|
}
|