mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
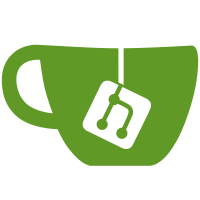
This piece of tidying-up has come out particularly well in terms of saving tedious repetition and boilerplate. I've managed to remove three pointless methods from every MAC implementation by means of writing them once centrally in terms of the implementation-specific methods; another method (hmacmd5_sink) vanished because I was able to make the interface type 'ssh2_mac' be directly usable as a BinarySink by way of a new delegation system; and because all the method implementations can now find their own vtable, I was even able to merge a lot of keying and output functions that had previously differed only in length parameters by having them look up the lengths in whatever vtable they were passed.
43 lines
1.1 KiB
C
43 lines
1.1 KiB
C
/*
|
|
* Centralised parts of the SSH-2 MAC API, which don't need to vary
|
|
* with the MAC implementation.
|
|
*/
|
|
|
|
#include <assert.h>
|
|
|
|
#include "ssh.h"
|
|
|
|
int ssh2_mac_verresult(ssh2_mac *mac, const void *candidate)
|
|
{
|
|
unsigned char correct[64]; /* at least as big as all known MACs */
|
|
int toret;
|
|
|
|
assert(mac->vt->len <= sizeof(correct));
|
|
ssh2_mac_genresult(mac, correct);
|
|
toret = smemeq(correct, candidate, mac->vt->len);
|
|
|
|
smemclr(correct, sizeof(correct));
|
|
|
|
return toret;
|
|
}
|
|
|
|
static void ssh2_mac_prepare(ssh2_mac *mac, const void *blk, int len,
|
|
unsigned long seq)
|
|
{
|
|
ssh2_mac_start(mac);
|
|
put_uint32(mac, seq);
|
|
put_data(mac, blk, len);
|
|
}
|
|
|
|
void ssh2_mac_generate(ssh2_mac *mac, void *blk, int len, unsigned long seq)
|
|
{
|
|
ssh2_mac_prepare(mac, blk, len, seq);
|
|
return ssh2_mac_genresult(mac, (unsigned char *)blk + len);
|
|
}
|
|
|
|
int ssh2_mac_verify(ssh2_mac *mac, const void *blk, int len, unsigned long seq)
|
|
{
|
|
ssh2_mac_prepare(mac, blk, len, seq);
|
|
return ssh2_mac_verresult(mac, (const unsigned char *)blk + len);
|
|
}
|