mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-01 11:32:48 -05:00
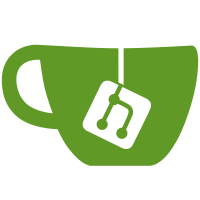
Various alignments I want to do in the host CA box have shown up deficiencies in this system, so I've reworked it a bit. Firstly, you can now specify more than two controls to be tied together with an align_next_to (e.g. multiple checkboxes alongside something else). Secondly, as well as forcing the controls to be the same height as each other, the layout algorithm will also move the later controls further _downward_, so that their top y positions also line up. Until now that hasn't been necessary, because they lined up already. In the GTK implementation of this via the Columns class, I've renamed 'columns_force_same_height' to 'columns_align_next_to', and similarly for some of the internal fields, since the latter change makes the previous names a misnomer. In the Windows implementation, I found it most convenient to set this up by following a linked list of align_next_to fields backwards. But it won't always be convenient to initialise them that way, so I've also written a crude normaliser that will rewrite those links into a canonical form. But I only call that on Windows; it's unnecessary in GTK, where the Columns class provides plenty of per-widget extra storage so I just keep each alignment class as a circular list.
74 lines
2.3 KiB
C
74 lines
2.3 KiB
C
/*
|
|
* columns.h - header file for a columns-based widget container
|
|
* capable of supporting the PuTTY portable dialog box layout
|
|
* mechanism.
|
|
*/
|
|
|
|
#ifndef COLUMNS_H
|
|
#define COLUMNS_H
|
|
|
|
#include <gdk/gdk.h>
|
|
#include <gtk/gtk.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif /* __cplusplus */
|
|
|
|
#define TYPE_COLUMNS (columns_get_type())
|
|
#define COLUMNS(obj) (G_TYPE_CHECK_INSTANCE_CAST((obj), TYPE_COLUMNS, Columns))
|
|
#define COLUMNS_CLASS(klass) \
|
|
(G_TYPE_CHECK_CLASS_CAST((klass), TYPE_COLUMNS, ColumnsClass))
|
|
#define IS_COLUMNS(obj) (G_TYPE_CHECK_INSTANCE_TYPE((obj), TYPE_COLUMNS))
|
|
#define IS_COLUMNS_CLASS(klass) (G_TYPE_CHECK_CLASS_TYPE((klass), TYPE_COLUMNS))
|
|
|
|
typedef struct Columns_tag Columns;
|
|
typedef struct ColumnsClass_tag ColumnsClass;
|
|
typedef struct ColumnsChild_tag ColumnsChild;
|
|
|
|
struct Columns_tag {
|
|
GtkContainer container;
|
|
/* private after here */
|
|
GList *children; /* this holds ColumnsChild structures */
|
|
GList *taborder; /* this just holds GtkWidgets */
|
|
ColumnsChild *vexpand;
|
|
gint spacing;
|
|
};
|
|
|
|
struct ColumnsClass_tag {
|
|
GtkContainerClass parent_class;
|
|
};
|
|
|
|
struct ColumnsChild_tag {
|
|
/* If `widget' is non-NULL, this entry represents an actual widget. */
|
|
GtkWidget *widget;
|
|
gint colstart, colspan;
|
|
bool force_left; /* for recalcitrant GtkLabels */
|
|
/* Otherwise, this entry represents a change in the column setup. */
|
|
gint ncols;
|
|
gint *percentages;
|
|
gint x, y, w, h; /* used during an individual size computation */
|
|
|
|
/* Circularly linked list of children that are vertically aligned
|
|
* with each other. */
|
|
ColumnsChild *valign_next, *valign_prev;
|
|
|
|
/* Temporary space used within some methods */
|
|
bool visited;
|
|
};
|
|
|
|
GType columns_get_type(void);
|
|
GtkWidget *columns_new(gint spacing);
|
|
void columns_set_cols(Columns *cols, gint ncols, const gint *percentages);
|
|
void columns_add(Columns *cols, GtkWidget *child,
|
|
gint colstart, gint colspan);
|
|
void columns_taborder_last(Columns *cols, GtkWidget *child);
|
|
void columns_force_left_align(Columns *cols, GtkWidget *child);
|
|
void columns_align_next_to(Columns *cols, GtkWidget *ch1, GtkWidget *ch2);
|
|
void columns_vexpand(Columns *cols, GtkWidget *child);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif /* __cplusplus */
|
|
|
|
#endif /* COLUMNS_H */
|