mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-05 05:22:47 -05:00
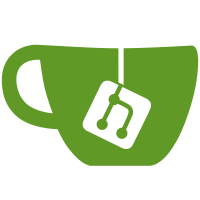
This parameter was undocumented, and Windows-specific: its semantics date from before PuTTY was cross-platform, and are "Pass this flags parameter straight through to the Win32 API's conversion functions". So in Windows platform code you can pass flags like MB_USEGLYPHCHARS, but in cross-platform code, you dare not pass anything nonzero at all because the Unix frontend won't recognise it (or, likely, even compile). I've kept the flag for now in the underlying mb_to_wc / wc_to_mb functions. Partly that's because there's one place in the Windows code where the parameter _is_ used; mostly, it's because I'm about to replace those functions anyway, so there's no point in editing all the call sites twice.
44 lines
1.2 KiB
C
44 lines
1.2 KiB
C
/*
|
|
* dup_wc_to_mb: memory-allocating wrapper on wc_to_mb.
|
|
*
|
|
* Also dup_wc_to_mb_c: same but you already know the length of the
|
|
* wide string, and you get told the length of the returned string.
|
|
* (But it's still NUL-terminated, for convenience.).
|
|
*/
|
|
|
|
#include <wchar.h>
|
|
|
|
#include "putty.h"
|
|
#include "misc.h"
|
|
|
|
char *dup_wc_to_mb_c(int codepage, const wchar_t *string,
|
|
size_t inlen, const char *defchr, size_t *outlen_p)
|
|
{
|
|
assert(inlen <= INT_MAX);
|
|
|
|
size_t outsize = inlen+1;
|
|
char *out = snewn(outsize, char);
|
|
|
|
while (true) {
|
|
size_t outlen = wc_to_mb(codepage, 0, string, inlen, out, outsize,
|
|
defchr);
|
|
/* We can only be sure we've consumed the whole input if the
|
|
* output is not within a multibyte-character-length of the
|
|
* end of the buffer! */
|
|
if (outlen < outsize && outsize - outlen > MB_LEN_MAX) {
|
|
if (outlen_p)
|
|
*outlen_p = outlen;
|
|
out[outlen] = '\0';
|
|
return out;
|
|
}
|
|
|
|
sgrowarray(out, outsize, outsize);
|
|
}
|
|
}
|
|
|
|
char *dup_wc_to_mb(int codepage, const wchar_t *string,
|
|
const char *defchr)
|
|
{
|
|
return dup_wc_to_mb_c(codepage, string, wcslen(string), defchr, NULL);
|
|
}
|