mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
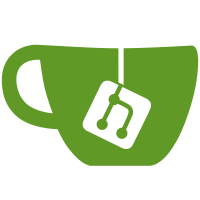
This is another cleanup I felt a need for while I was doing boolification. If you define a function or variable in one .c file and declare it extern in another, then nothing will check you haven't got the types of the two declarations mismatched - so when you're _changing_ the type, it's a pain to make sure you've caught all the copies of it. It's better to put all those extern declarations in header files, so that the declaration in the header is also in scope for the definition. Then the compiler will complain if they don't match, which is what I want.
69 lines
1.7 KiB
C
69 lines
1.7 KiB
C
/*
|
|
* EC key generation.
|
|
*/
|
|
|
|
#include "ssh.h"
|
|
|
|
int ec_generate(struct ec_key *key, int bits, progfn_t pfn,
|
|
void *pfnparam)
|
|
{
|
|
struct ec_point *publicKey;
|
|
|
|
if (!ec_nist_alg_and_curve_by_bits(bits, &key->publicKey.curve,
|
|
&key->sshk))
|
|
return 0;
|
|
|
|
key->privateKey = bignum_random_in_range(One, key->publicKey.curve->w.n);
|
|
if (!key->privateKey) return 0;
|
|
|
|
publicKey = ec_public(key->privateKey, key->publicKey.curve);
|
|
if (!publicKey) {
|
|
freebn(key->privateKey);
|
|
key->privateKey = NULL;
|
|
return 0;
|
|
}
|
|
|
|
key->publicKey.x = publicKey->x;
|
|
key->publicKey.y = publicKey->y;
|
|
key->publicKey.z = NULL;
|
|
sfree(publicKey);
|
|
|
|
return 1;
|
|
}
|
|
|
|
int ec_edgenerate(struct ec_key *key, int bits, progfn_t pfn,
|
|
void *pfnparam)
|
|
{
|
|
struct ec_point *publicKey;
|
|
|
|
if (!ec_ed_alg_and_curve_by_bits(bits, &key->publicKey.curve,
|
|
&key->sshk))
|
|
return 0;
|
|
|
|
{
|
|
/* EdDSA secret keys are just 32 bytes of hash preimage; the
|
|
* 64-byte SHA-512 hash of that key will be used when signing,
|
|
* but the form of the key stored on disk is the preimage
|
|
* only. */
|
|
Bignum privMax = bn_power_2(bits);
|
|
if (!privMax) return 0;
|
|
key->privateKey = bignum_random_in_range(Zero, privMax);
|
|
freebn(privMax);
|
|
if (!key->privateKey) return 0;
|
|
}
|
|
|
|
publicKey = ec_public(key->privateKey, key->publicKey.curve);
|
|
if (!publicKey) {
|
|
freebn(key->privateKey);
|
|
key->privateKey = NULL;
|
|
return 0;
|
|
}
|
|
|
|
key->publicKey.x = publicKey->x;
|
|
key->publicKey.y = publicKey->y;
|
|
key->publicKey.z = NULL;
|
|
sfree(publicKey);
|
|
|
|
return 1;
|
|
}
|