mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
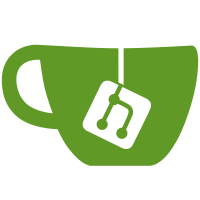
When anyone connects to a PuTTY tool's listening socket - whether it's a user of a local->remote port forwarding, a connection-sharing downstream or a client of Pageant - we'd like to log as much information as we can find out about where the connection came from. To that end, I've implemented a function sk_peer_info() in the socket abstraction, which returns a freeform text string as best it can (or NULL, if it can't get anything at all) describing the thing at the other end of the connection. For TCP connections, this is done using getpeername() to get an IP address and port in the obvious way; for Unix-domain sockets, we attempt SO_PEERCRED (conditionalised on some moderately hairy autoconfery) to get the pid and owner of the peer. I haven't implemented anything for Windows named pipes, but I will if I hear of anything useful.
74 lines
1.4 KiB
C
74 lines
1.4 KiB
C
/*
|
|
* A dummy Socket implementation which just holds an error message.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <assert.h>
|
|
|
|
#define DEFINE_PLUG_METHOD_MACROS
|
|
#include "tree234.h"
|
|
#include "putty.h"
|
|
#include "network.h"
|
|
|
|
typedef struct Socket_error_tag *Error_Socket;
|
|
|
|
struct Socket_error_tag {
|
|
const struct socket_function_table *fn;
|
|
/* the above variable absolutely *must* be the first in this structure */
|
|
|
|
char *error;
|
|
Plug plug;
|
|
};
|
|
|
|
static Plug sk_error_plug(Socket s, Plug p)
|
|
{
|
|
Error_Socket ps = (Error_Socket) s;
|
|
Plug ret = ps->plug;
|
|
if (p)
|
|
ps->plug = p;
|
|
return ret;
|
|
}
|
|
|
|
static void sk_error_close(Socket s)
|
|
{
|
|
Error_Socket ps = (Error_Socket) s;
|
|
|
|
sfree(ps->error);
|
|
sfree(ps);
|
|
}
|
|
|
|
static const char *sk_error_socket_error(Socket s)
|
|
{
|
|
Error_Socket ps = (Error_Socket) s;
|
|
return ps->error;
|
|
}
|
|
|
|
static char *sk_error_peer_info(Socket s)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
Socket new_error_socket(const char *errmsg, Plug plug)
|
|
{
|
|
static const struct socket_function_table socket_fn_table = {
|
|
sk_error_plug,
|
|
sk_error_close,
|
|
NULL /* write */,
|
|
NULL /* write_oob */,
|
|
NULL /* write_eof */,
|
|
NULL /* flush */,
|
|
NULL /* set_frozen */,
|
|
sk_error_socket_error,
|
|
sk_error_peer_info,
|
|
};
|
|
|
|
Error_Socket ret;
|
|
|
|
ret = snew(struct Socket_error_tag);
|
|
ret->fn = &socket_fn_table;
|
|
ret->plug = plug;
|
|
ret->error = dupstr(errmsg);
|
|
|
|
return (Socket) ret;
|
|
}
|