mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-08 23:03:43 -05:00
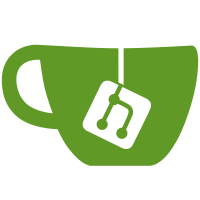
In a GUI app, when interactive userpass input begins, the Ldisc acquires a reference to a prompts_t. If something bad happens to the SSH connection (e.g. unexpected server-side closure), then all the SSH layers will be destroyed, including freeing that prompts_t. So the Ldisc will have a stale reference to it, which it might potentially use. To fix that, I've arranged a back-pointer so that prompts_t itself can find the Ldisc's reference to it, and NULL it out on free. So now, whichever of a prompts_t and an Ldisc is freed first, the link between them should be cleanly broken. (I'm not 100% sure this is absolutely necessary, in the sense of whether a sequence of events can _actually_ happen that causes a stale pointer dereference. But I don't want to take the chance!)
70 lines
1.5 KiB
C
70 lines
1.5 KiB
C
/*
|
|
* Functions for making, destroying, and manipulating prompts_t
|
|
* structures.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
prompts_t *new_prompts(void)
|
|
{
|
|
prompts_t *p = snew(prompts_t);
|
|
p->prompts = NULL;
|
|
p->n_prompts = p->prompts_size = 0;
|
|
p->data = NULL;
|
|
p->idata = -1;
|
|
p->to_server = true; /* to be on the safe side */
|
|
p->name = p->instruction = NULL;
|
|
p->name_reqd = p->instr_reqd = false;
|
|
p->callback = NULL;
|
|
p->callback_ctx = NULL;
|
|
p->ldisc_ptr_to_us = NULL;
|
|
return p;
|
|
}
|
|
|
|
void add_prompt(prompts_t *p, char *promptstr, bool echo)
|
|
{
|
|
prompt_t *pr = snew(prompt_t);
|
|
pr->prompt = promptstr;
|
|
pr->echo = echo;
|
|
pr->result = strbuf_new_nm();
|
|
sgrowarray(p->prompts, p->prompts_size, p->n_prompts);
|
|
p->prompts[p->n_prompts++] = pr;
|
|
}
|
|
|
|
void prompt_set_result(prompt_t *pr, const char *newstr)
|
|
{
|
|
strbuf_clear(pr->result);
|
|
put_datapl(pr->result, ptrlen_from_asciz(newstr));
|
|
}
|
|
|
|
const char *prompt_get_result_ref(prompt_t *pr)
|
|
{
|
|
return pr->result->s;
|
|
}
|
|
|
|
char *prompt_get_result(prompt_t *pr)
|
|
{
|
|
return dupstr(pr->result->s);
|
|
}
|
|
|
|
void free_prompts(prompts_t *p)
|
|
{
|
|
size_t i;
|
|
|
|
/* If an Ldisc currently knows about us, tell it to forget us, so
|
|
* it won't dereference a stale pointer later. */
|
|
if (p->ldisc_ptr_to_us)
|
|
*p->ldisc_ptr_to_us = NULL;
|
|
|
|
for (i=0; i < p->n_prompts; i++) {
|
|
prompt_t *pr = p->prompts[i];
|
|
strbuf_free(pr->result);
|
|
sfree(pr->prompt);
|
|
sfree(pr);
|
|
}
|
|
sfree(p->prompts);
|
|
sfree(p->name);
|
|
sfree(p->instruction);
|
|
sfree(p);
|
|
}
|