mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 17:38:00 +00:00
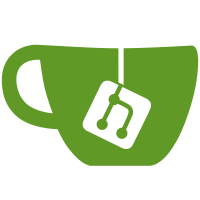
malloc functions, which automatically cast to the same type they're allocating the size of. Should prevent any future errors involving mallocing the size of the wrong structure type, and will also make life easier if we ever need to turn the PuTTY core code from real C into C++-friendly C. I haven't touched the Mac frontend in this checkin because I couldn't compile or test it. [originally from svn r3014]
39 lines
1.1 KiB
C
39 lines
1.1 KiB
C
/*
|
|
* PuTTY memory-handling header.
|
|
*/
|
|
|
|
#ifndef PUTTY_PUTTYMEM_H
|
|
#define PUTTY_PUTTYMEM_H
|
|
|
|
#include <stddef.h> /* for size_t */
|
|
#include <string.h> /* for memcpy() */
|
|
|
|
|
|
/* #define MALLOC_LOG do this if you suspect putty of leaking memory */
|
|
#ifdef MALLOC_LOG
|
|
#define smalloc(z) (mlog(__FILE__,__LINE__), safemalloc(z))
|
|
#define srealloc(y,z) (mlog(__FILE__,__LINE__), saferealloc(y,z))
|
|
#define sfree(z) (mlog(__FILE__,__LINE__), safefree(z))
|
|
void mlog(char *, int);
|
|
#else
|
|
#define smalloc safemalloc
|
|
#define srealloc saferealloc
|
|
#define sfree safefree
|
|
#endif
|
|
|
|
void *safemalloc(size_t);
|
|
void *saferealloc(void *, size_t);
|
|
void safefree(void *);
|
|
|
|
/*
|
|
* Direct use of smalloc within the code should be avoided where
|
|
* possible, in favour of these type-casting macros which ensure
|
|
* you don't mistakenly allocate enough space for one sort of
|
|
* structure and assign it to a different sort of pointer.
|
|
*/
|
|
#define snew(type) ((type *)smalloc(sizeof(type)))
|
|
#define snewn(n, type) ((type *)smalloc((n)*sizeof(type)))
|
|
#define sresize(ptr, n, type) ((type *)srealloc(ptr, (n)*sizeof(type)))
|
|
|
|
#endif
|