mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
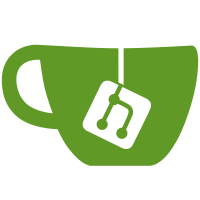
This is working towards allowing the subsidiary SSH connection in an SshProxy to share the main user-facing Seat, so as to be able to pass through interactive prompts. This is more difficult than the similar change with LogPolicy, because Seats are stateful. In particular, the trust-sigil status will need to be controlled by the SshProxy until it's ready to pass over control to the main SSH (or whatever) connection. To make this work, I've introduced a thing called a TempSeat, which is (yet) another Seat implementation. When a backend hands its Seat to new_connection(), it does it in a way that allows new_connection() to borrow it completely, and replace it in the main backend structure with a TempSeat, which acts as a temporary placeholder. If the main backend tries to do things like changing trust status or sending output, the TempSeat will buffer them; later on, when the connection is established, TempSeat will replay the changes into the real Seat. So, in each backend, I've made the following changes: - pass &foo->seat to new_connection, which may overwrite it with a TempSeat. - if it has done so (which we can tell via the is_tempseat() query function), then we have to free the TempSeat and reinstate our main Seat. The signal that we can do so is the PLUGLOG_CONNECT_SUCCESS notification, which indicates that SshProxy has finished all its connection setup work. - we also have to remember to free the TempSeat if our backend is disposed of without that having happened (e.g. because the connection _doesn't_ succeed). - in backends which have no local auth phase to worry about, ensure we don't call seat_set_trust_status on the main Seat _before_ it gets potentially replaced with a TempSeat. Moved some calls of seat_set_trust_status to just after new_connection(), so that now the initial trust status setup will go into the TempSeat (if appropriate) and be buffered until that seat is relinquished. In all other uses of new_connection, where we don't have a Seat available at all, we just pass NULL. This is NFC, because neither new_connection() nor any of its delegates will _actually_ do this replacement yet. We're just setting up the framework to enable it to do so in the next commit.
33 lines
1.2 KiB
C
33 lines
1.2 KiB
C
/*
|
|
* noproxy.c: an alternative to proxy.c, for use by auxiliary programs
|
|
* that need to make network connections but don't want to include all
|
|
* the full-on support for endless network proxies (and its
|
|
* configuration requirements). Implements the primary APIs of
|
|
* proxy.c, but maps them straight to the underlying network layer.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
#include "network.h"
|
|
#include "proxy.h"
|
|
|
|
SockAddr *name_lookup(const char *host, int port, char **canonicalname,
|
|
Conf *conf, int addressfamily, LogContext *logctx,
|
|
const char *reason)
|
|
{
|
|
return sk_namelookup(host, canonicalname, addressfamily);
|
|
}
|
|
|
|
Socket *new_connection(SockAddr *addr, const char *hostname,
|
|
int port, bool privport,
|
|
bool oobinline, bool nodelay, bool keepalive,
|
|
Plug *plug, Conf *conf, LogPolicy *lp, Seat **seat)
|
|
{
|
|
return sk_new(addr, port, privport, oobinline, nodelay, keepalive, plug);
|
|
}
|
|
|
|
Socket *new_listener(const char *srcaddr, int port, Plug *plug,
|
|
bool local_host_only, Conf *conf, int addressfamily)
|
|
{
|
|
return sk_newlistener(srcaddr, port, plug, local_host_only, addressfamily);
|
|
}
|