mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-08 08:58:00 +00:00
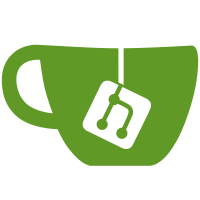
This is built more or less entirely out of pieces I already had. The SOCKS server code is provided by the dynamic forwarding code in portfwd.c. When that accepts a connection request, it wants to talk to an SSH ConnectionLayer, which is already a trait with interchangeable implementations - so I just provide one of my own which only supports the lportfwd_open() method. And that in turn returns an SshChannel object, with a special trait implementation all of whose methods just funnel back to an ordinary Socket. Result: you get a Socket-to-Socket SOCKS implementation with no SSH anywhere, and even a minimal amount of need to _pretend_ internally to be an SSH implementation. Additional features include the ability to log all the traffic in the form of diagnostics to standard error, or log each direction of each connection separately to a file, or for anything more general, to log each direction of each connection through a pipe to a subcommand that can filter out whatever you think are the interesting parts. Also, you can spawn a subcommand after the SOCKS server is set up, and terminate automatically when that subcommand does - e.g. you might use this to wrap the execution of a single SOCKS-using program. This is a modernisation of a diagnostic utility I've had kicking around out-of-tree for a long time. With all of last year's refactorings, it now becomes feasible to keep it in-tree without needing huge amounts of scaffolding. Also, this version runs on Windows, which is more than the old one did. (On Windows I haven't implemented the subprocess parts, although there's no reason I _couldn't_.) As well as diagnostic uses, this may also be useful in some situations as a thing to forward ports to: PuTTY doesn't currently support reverse dynamic port forwarding (in which the remote listening port acts as a SOCKS server), but you could get the same effect by forwarding a remote port to a local instance of this. (Although, of course, that's nothing you couldn't achieve using any other SOCKS server.)
29 lines
850 B
C
29 lines
850 B
C
typedef struct psocks_state psocks_state;
|
|
|
|
typedef struct PsocksPlatform PsocksPlatform;
|
|
typedef struct PsocksDataSink PsocksDataSink;
|
|
|
|
/* indices into PsocksDataSink arrays */
|
|
typedef enum PsocksDirection { UP, DN } PsocksDirection;
|
|
|
|
typedef struct PsocksDataSink {
|
|
void (*free)(PsocksDataSink *);
|
|
BinarySink *s[2];
|
|
} PsocksDataSink;
|
|
static inline void pds_free(PsocksDataSink *pds)
|
|
{ pds->free(pds); }
|
|
|
|
PsocksDataSink *pds_stdio(FILE *fp[2]);
|
|
|
|
struct PsocksPlatform {
|
|
PsocksDataSink *(*open_pipes)(
|
|
const char *cmd, const char *const *direction_args,
|
|
const char *index_arg, char **err);
|
|
void (*start_subcommand)(strbuf *args);
|
|
};
|
|
|
|
psocks_state *psocks_new(const PsocksPlatform *);
|
|
void psocks_free(psocks_state *ps);
|
|
void psocks_cmdline(psocks_state *ps, int argc, char **argv);
|
|
void psocks_start(psocks_state *ps);
|