mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-17 11:00:59 -05:00
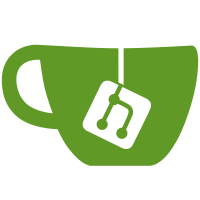
DIT, for 'Data-Independent Timing', is a bit you can set in the processor state on sufficiently new Arm CPUs, which promises that a long list of instructions will deliberately avoid varying their timing based on the input register values. Just what you want for keeping your constant-time crypto primitives constant-time. As far as I'm aware, no CPU has _yet_ implemented any data-dependent optimisations, so DIT is a safety precaution against them doing so in future. It would be embarrassing to be caught without it if a future CPU does do that, so we now turn on DIT in the PuTTY process state. I've put a call to the new enable_dit() function at the start of every main() and WinMain() belonging to a program that might do cryptography (even testcrypt, in case someone uses it for something!), and in case I missed one there, also added a second call at the first moment that any cryptography-using part of the code looks as if it might become active: when an instance of the SSH protocol object is configured, when the system PRNG is initialised, and when selecting any cryptographic authentication protocol in an HTTP or SOCKS proxy connection. With any luck those precautions between them should ensure it's on whenever we need it. Arm's own recommendation is that you should carefully choose the granularity at which you enable and disable DIT: there's a potential time cost to turning it on and off (I'm not sure what, but plausibly something of the order of a pipeline flush), so it's a performance hit to do it _inside_ each individual crypto function, but if CPUs start supporting significant data-dependent optimisation in future, then it will also become a noticeable performance hit to just leave it on across the whole process. So you'd like to do it somewhere in the middle: for example, you might turn on DIT once around the whole process of verifying and decrypting an SSH packet, instead of once for decryption and once for MAC. With all respect to that recommendation as a strategy for maximum performance, I'm not following it here. I turn on DIT at the start of the PuTTY process, and then leave it on. Rationale: 1. PuTTY is not otherwise a performance-critical application: it's not likely to max out your CPU for any purpose _other_ than cryptography. The most CPU-intensive non-cryptographic thing I can imagine a PuTTY process doing is the complicated computation of font rendering in the terminal, and that will normally be cached (you don't recompute each glyph from its outline and hints for every time you display it). 2. I think a bigger risk lies in accidental side channels from having DIT turned off when it should have been on. I can imagine lots of causes for that. Missing a crypto operation in some unswept corner of the code; confusing control flow (like my coroutine macros) jumping with DIT clear into the middle of a region of code that expected DIT to have been set at the beginning; having a reference counter of DIT requests and getting it out of sync. In a more sophisticated programming language, it might be possible to avoid the risk in #2 by cleverness with the type system. For example, in Rust, you could have a zero-sized type that acts as a proof token for DIT being enabled (it would be constructed by a function that also sets DIT, have a Drop implementation that clears DIT, and be !Send so you couldn't use it in a thread other than the one where DIT was set), and then you could require all the actual crypto functions to take a DitToken as an extra parameter, at zero runtime cost. Then "oops I forgot to set DIT around this piece of crypto" would become a compile error. Even so, you'd have to take some care with coroutine-structured code (what happens if a Rust async function yields while holding a DIT token?) and with nesting (if you have two DIT tokens, you don't want dropping the inner one to clear DIT while the outer one is still there to wrongly convince callees that it's set). Maybe in Rust you could get this all to work reliably. But not in C! DIT is an optional feature of the Arm architecture, so we must first test to see if it's supported. This is done the same way as we already do for the various Arm crypto accelerators: on ELF-based systems, check the appropriate bit in the 'hwcap' words in the ELF aux vector; on Mac, look for an appropriate sysctl flag. On Windows I don't know of a way to query the DIT feature, _or_ of a way to write the necessary enabling instruction in an MSVC-compatible way. I've _heard_ that it might not be necessary, because Windows might just turn on DIT unconditionally and leave it on, in an even more extreme version of my own strategy. I don't have a source for that - I heard it by word of mouth - but I _hope_ it's true, because that would suit me very well! Certainly I can't write code to enable DIT without knowing (a) how to do it, (b) how to know if it's safe. Nonetheless, I've put the enable_dit() call in all the right places in the Windows main programs as well as the Unix and cross-platform code, so that if I later find out that I _can_ put in an explicit enable of DIT in some way, I'll only have to arrange to set HAVE_ARM_DIT and compile the enable_dit() function appropriately.
584 lines
12 KiB
C
584 lines
12 KiB
C
/*
|
|
* sftp.c: the Unix-specific parts of PSFTP and PSCP.
|
|
*/
|
|
|
|
#include <sys/time.h>
|
|
#include <sys/types.h>
|
|
#include <sys/stat.h>
|
|
#include <stdlib.h>
|
|
#include <fcntl.h>
|
|
#include <dirent.h>
|
|
#include <unistd.h>
|
|
#include <utime.h>
|
|
#include <errno.h>
|
|
#include <assert.h>
|
|
|
|
#include "putty.h"
|
|
#include "ssh.h"
|
|
#include "psftp.h"
|
|
|
|
#if HAVE_GLOB_H
|
|
#include <glob.h>
|
|
#endif
|
|
|
|
char *x_get_default(const char *key)
|
|
{
|
|
return NULL; /* this is a stub */
|
|
}
|
|
|
|
void platform_get_x11_auth(struct X11Display *display, Conf *conf)
|
|
{
|
|
/* Do nothing, therefore no auth. */
|
|
}
|
|
const bool platform_uses_x11_unix_by_default = true;
|
|
|
|
/*
|
|
* Default settings that are specific to PSFTP.
|
|
*/
|
|
char *platform_default_s(const char *name)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
bool platform_default_b(const char *name, bool def)
|
|
{
|
|
return def;
|
|
}
|
|
|
|
int platform_default_i(const char *name, int def)
|
|
{
|
|
return def;
|
|
}
|
|
|
|
FontSpec *platform_default_fontspec(const char *name)
|
|
{
|
|
return fontspec_new_default();
|
|
}
|
|
|
|
Filename *platform_default_filename(const char *name)
|
|
{
|
|
if (!strcmp(name, "LogFileName"))
|
|
return filename_from_str("putty.log");
|
|
else
|
|
return filename_from_str("");
|
|
}
|
|
|
|
SeatPromptResult filexfer_get_userpass_input(Seat *seat, prompts_t *p)
|
|
{
|
|
/* The file transfer tools don't support Restart Session, so we
|
|
* can just have a single static cmdline_get_passwd_input_state
|
|
* that's never reset */
|
|
static cmdline_get_passwd_input_state cmdline_state =
|
|
CMDLINE_GET_PASSWD_INPUT_STATE_INIT;
|
|
|
|
SeatPromptResult spr;
|
|
spr = cmdline_get_passwd_input(p, &cmdline_state, false);
|
|
if (spr.kind == SPRK_INCOMPLETE)
|
|
spr = console_get_userpass_input(p);
|
|
return spr;
|
|
}
|
|
|
|
/*
|
|
* Set local current directory. Returns NULL on success, or else an
|
|
* error message which must be freed after printing.
|
|
*/
|
|
char *psftp_lcd(char *dir)
|
|
{
|
|
if (chdir(dir) < 0)
|
|
return dupprintf("%s: chdir: %s", dir, strerror(errno));
|
|
else
|
|
return NULL;
|
|
}
|
|
|
|
/*
|
|
* Get local current directory. Returns a string which must be
|
|
* freed.
|
|
*/
|
|
char *psftp_getcwd(void)
|
|
{
|
|
char *buffer, *ret;
|
|
size_t size = 256;
|
|
|
|
buffer = snewn(size, char);
|
|
while (1) {
|
|
ret = getcwd(buffer, size);
|
|
if (ret != NULL)
|
|
return ret;
|
|
if (errno != ERANGE) {
|
|
sfree(buffer);
|
|
return dupprintf("[cwd unavailable: %s]", strerror(errno));
|
|
}
|
|
/*
|
|
* Otherwise, ERANGE was returned, meaning the buffer
|
|
* wasn't big enough.
|
|
*/
|
|
sgrowarray(buffer, size, size);
|
|
}
|
|
}
|
|
|
|
struct RFile {
|
|
int fd;
|
|
};
|
|
|
|
RFile *open_existing_file(const char *name, uint64_t *size,
|
|
unsigned long *mtime, unsigned long *atime,
|
|
long *perms)
|
|
{
|
|
int fd;
|
|
RFile *f;
|
|
|
|
fd = open(name, O_RDONLY);
|
|
if (fd < 0)
|
|
return NULL;
|
|
|
|
f = snew(RFile);
|
|
f->fd = fd;
|
|
|
|
if (size || mtime || atime || perms) {
|
|
struct stat statbuf;
|
|
if (fstat(fd, &statbuf) < 0) {
|
|
fprintf(stderr, "%s: stat: %s\n", name, strerror(errno));
|
|
memset(&statbuf, 0, sizeof(statbuf));
|
|
}
|
|
|
|
if (size)
|
|
*size = statbuf.st_size;
|
|
|
|
if (mtime)
|
|
*mtime = statbuf.st_mtime;
|
|
|
|
if (atime)
|
|
*atime = statbuf.st_atime;
|
|
|
|
if (perms)
|
|
*perms = statbuf.st_mode;
|
|
}
|
|
|
|
return f;
|
|
}
|
|
|
|
int read_from_file(RFile *f, void *buffer, int length)
|
|
{
|
|
return read(f->fd, buffer, length);
|
|
}
|
|
|
|
void close_rfile(RFile *f)
|
|
{
|
|
close(f->fd);
|
|
sfree(f);
|
|
}
|
|
|
|
struct WFile {
|
|
int fd;
|
|
char *name;
|
|
};
|
|
|
|
WFile *open_new_file(const char *name, long perms)
|
|
{
|
|
int fd;
|
|
WFile *f;
|
|
|
|
fd = open(name, O_CREAT | O_TRUNC | O_WRONLY,
|
|
(mode_t)(perms ? perms : 0666));
|
|
if (fd < 0)
|
|
return NULL;
|
|
|
|
f = snew(WFile);
|
|
f->fd = fd;
|
|
f->name = dupstr(name);
|
|
|
|
return f;
|
|
}
|
|
|
|
|
|
WFile *open_existing_wfile(const char *name, uint64_t *size)
|
|
{
|
|
int fd;
|
|
WFile *f;
|
|
|
|
fd = open(name, O_APPEND | O_WRONLY);
|
|
if (fd < 0)
|
|
return NULL;
|
|
|
|
f = snew(WFile);
|
|
f->fd = fd;
|
|
f->name = dupstr(name);
|
|
|
|
if (size) {
|
|
struct stat statbuf;
|
|
if (fstat(fd, &statbuf) < 0) {
|
|
fprintf(stderr, "%s: stat: %s\n", name, strerror(errno));
|
|
memset(&statbuf, 0, sizeof(statbuf));
|
|
}
|
|
|
|
*size = statbuf.st_size;
|
|
}
|
|
|
|
return f;
|
|
}
|
|
|
|
int write_to_file(WFile *f, void *buffer, int length)
|
|
{
|
|
char *p = (char *)buffer;
|
|
int so_far = 0;
|
|
|
|
/* Keep trying until we've really written as much as we can. */
|
|
while (length > 0) {
|
|
int ret = write(f->fd, p, length);
|
|
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
if (ret == 0)
|
|
break;
|
|
|
|
p += ret;
|
|
length -= ret;
|
|
so_far += ret;
|
|
}
|
|
|
|
return so_far;
|
|
}
|
|
|
|
void set_file_times(WFile *f, unsigned long mtime, unsigned long atime)
|
|
{
|
|
struct utimbuf ut;
|
|
|
|
ut.actime = atime;
|
|
ut.modtime = mtime;
|
|
|
|
utime(f->name, &ut);
|
|
}
|
|
|
|
/* Closes and frees the WFile */
|
|
void close_wfile(WFile *f)
|
|
{
|
|
close(f->fd);
|
|
sfree(f->name);
|
|
sfree(f);
|
|
}
|
|
|
|
/* Seek offset bytes through file, from whence, where whence is
|
|
FROM_START, FROM_CURRENT, or FROM_END */
|
|
int seek_file(WFile *f, uint64_t offset, int whence)
|
|
{
|
|
int lseek_whence;
|
|
|
|
switch (whence) {
|
|
case FROM_START:
|
|
lseek_whence = SEEK_SET;
|
|
break;
|
|
case FROM_CURRENT:
|
|
lseek_whence = SEEK_CUR;
|
|
break;
|
|
case FROM_END:
|
|
lseek_whence = SEEK_END;
|
|
break;
|
|
default:
|
|
return -1;
|
|
}
|
|
|
|
return lseek(f->fd, offset, lseek_whence) >= 0 ? 0 : -1;
|
|
}
|
|
|
|
uint64_t get_file_posn(WFile *f)
|
|
{
|
|
return lseek(f->fd, (off_t) 0, SEEK_CUR);
|
|
}
|
|
|
|
int file_type(const char *name)
|
|
{
|
|
struct stat statbuf;
|
|
|
|
if (stat(name, &statbuf) < 0) {
|
|
if (errno != ENOENT)
|
|
fprintf(stderr, "%s: stat: %s\n", name, strerror(errno));
|
|
return FILE_TYPE_NONEXISTENT;
|
|
}
|
|
|
|
if (S_ISREG(statbuf.st_mode))
|
|
return FILE_TYPE_FILE;
|
|
|
|
if (S_ISDIR(statbuf.st_mode))
|
|
return FILE_TYPE_DIRECTORY;
|
|
|
|
return FILE_TYPE_WEIRD;
|
|
}
|
|
|
|
struct DirHandle {
|
|
DIR *dir;
|
|
};
|
|
|
|
DirHandle *open_directory(const char *name, const char **errmsg)
|
|
{
|
|
DIR *dp = opendir(name);
|
|
if (!dp) {
|
|
*errmsg = strerror(errno);
|
|
return NULL;
|
|
}
|
|
|
|
DirHandle *dir = snew(DirHandle);
|
|
dir->dir = dp;
|
|
return dir;
|
|
}
|
|
|
|
char *read_filename(DirHandle *dir)
|
|
{
|
|
struct dirent *de;
|
|
|
|
do {
|
|
de = readdir(dir->dir);
|
|
if (de == NULL)
|
|
return NULL;
|
|
} while ((de->d_name[0] == '.' &&
|
|
(de->d_name[1] == '\0' ||
|
|
(de->d_name[1] == '.' && de->d_name[2] == '\0'))));
|
|
|
|
return dupstr(de->d_name);
|
|
}
|
|
|
|
void close_directory(DirHandle *dir)
|
|
{
|
|
closedir(dir->dir);
|
|
sfree(dir);
|
|
}
|
|
|
|
int test_wildcard(const char *name, bool cmdline)
|
|
{
|
|
struct stat statbuf;
|
|
|
|
if (stat(name, &statbuf) == 0) {
|
|
return WCTYPE_FILENAME;
|
|
} else if (cmdline) {
|
|
/*
|
|
* On Unix, we never need to parse wildcards coming from
|
|
* the command line, because the shell will have expanded
|
|
* them into a filename list already.
|
|
*/
|
|
return WCTYPE_NONEXISTENT;
|
|
} else {
|
|
#if HAVE_GLOB_H
|
|
glob_t globbed;
|
|
int ret = WCTYPE_NONEXISTENT;
|
|
|
|
if (glob(name, GLOB_ERR, NULL, &globbed) == 0) {
|
|
if (globbed.gl_pathc > 0)
|
|
ret = WCTYPE_WILDCARD;
|
|
globfree(&globbed);
|
|
}
|
|
|
|
return ret;
|
|
#else
|
|
/* On a system without glob.h, we just have to return a
|
|
* failure code */
|
|
return WCTYPE_NONEXISTENT;
|
|
#endif
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Actually return matching file names for a local wildcard.
|
|
*/
|
|
#if HAVE_GLOB_H
|
|
struct WildcardMatcher {
|
|
glob_t globbed;
|
|
int i;
|
|
};
|
|
WildcardMatcher *begin_wildcard_matching(const char *name) {
|
|
WildcardMatcher *dir = snew(WildcardMatcher);
|
|
|
|
if (glob(name, 0, NULL, &dir->globbed) < 0) {
|
|
sfree(dir);
|
|
return NULL;
|
|
}
|
|
|
|
dir->i = 0;
|
|
|
|
return dir;
|
|
}
|
|
char *wildcard_get_filename(WildcardMatcher *dir) {
|
|
if (dir->i < dir->globbed.gl_pathc) {
|
|
return dupstr(dir->globbed.gl_pathv[dir->i++]);
|
|
} else
|
|
return NULL;
|
|
}
|
|
void finish_wildcard_matching(WildcardMatcher *dir) {
|
|
globfree(&dir->globbed);
|
|
sfree(dir);
|
|
}
|
|
#else
|
|
WildcardMatcher *begin_wildcard_matching(const char *name)
|
|
{
|
|
return NULL;
|
|
}
|
|
char *wildcard_get_filename(WildcardMatcher *dir)
|
|
{
|
|
unreachable("Can't construct a valid WildcardMatcher without <glob.h>");
|
|
}
|
|
void finish_wildcard_matching(WildcardMatcher *dir)
|
|
{
|
|
unreachable("Can't construct a valid WildcardMatcher without <glob.h>");
|
|
}
|
|
#endif
|
|
|
|
char *stripslashes(const char *str, bool local)
|
|
{
|
|
char *p;
|
|
|
|
/*
|
|
* On Unix, we do the same thing regardless of the 'local'
|
|
* parameter.
|
|
*/
|
|
p = strrchr(str, '/');
|
|
if (p) str = p+1;
|
|
|
|
return (char *)str;
|
|
}
|
|
|
|
bool vet_filename(const char *name)
|
|
{
|
|
if (strchr(name, '/'))
|
|
return false;
|
|
|
|
if (name[0] == '.' && (!name[1] || (name[1] == '.' && !name[2])))
|
|
return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
bool create_directory(const char *name)
|
|
{
|
|
return mkdir(name, 0777) == 0;
|
|
}
|
|
|
|
char *dir_file_cat(const char *dir, const char *file)
|
|
{
|
|
ptrlen dir_pl = ptrlen_from_asciz(dir);
|
|
return dupcat(
|
|
dir, ptrlen_endswith(dir_pl, PTRLEN_LITERAL("/"), NULL) ? "" : "/",
|
|
file);
|
|
}
|
|
|
|
/*
|
|
* Do a select() between all currently active network fds and
|
|
* optionally stdin, using cli_main_loop.
|
|
*/
|
|
|
|
struct ssh_sftp_mainloop_ctx {
|
|
bool include_stdin, no_fds_ok;
|
|
int toret;
|
|
};
|
|
static bool ssh_sftp_pw_setup(void *vctx, pollwrapper *pw)
|
|
{
|
|
struct ssh_sftp_mainloop_ctx *ctx = (struct ssh_sftp_mainloop_ctx *)vctx;
|
|
int fdstate, rwx;
|
|
|
|
if (!ctx->no_fds_ok && !toplevel_callback_pending() &&
|
|
first_fd(&fdstate, &rwx) < 0) {
|
|
ctx->toret = -1;
|
|
return false; /* terminate cli_main_loop */
|
|
}
|
|
|
|
if (ctx->include_stdin)
|
|
pollwrap_add_fd_rwx(pw, 0, SELECT_R);
|
|
|
|
return true;
|
|
}
|
|
static void ssh_sftp_pw_check(void *vctx, pollwrapper *pw)
|
|
{
|
|
struct ssh_sftp_mainloop_ctx *ctx = (struct ssh_sftp_mainloop_ctx *)vctx;
|
|
|
|
if (ctx->include_stdin && pollwrap_check_fd_rwx(pw, 0, SELECT_R))
|
|
ctx->toret = 1;
|
|
}
|
|
static bool ssh_sftp_mainloop_continue(void *vctx, bool found_any_fd,
|
|
bool ran_any_callback)
|
|
{
|
|
struct ssh_sftp_mainloop_ctx *ctx = (struct ssh_sftp_mainloop_ctx *)vctx;
|
|
if (ctx->toret != 0 || found_any_fd || ran_any_callback)
|
|
return false; /* finish the loop */
|
|
return true;
|
|
}
|
|
static int ssh_sftp_do_select(bool include_stdin, bool no_fds_ok)
|
|
{
|
|
struct ssh_sftp_mainloop_ctx ctx[1];
|
|
ctx->include_stdin = include_stdin;
|
|
ctx->no_fds_ok = no_fds_ok;
|
|
ctx->toret = 0;
|
|
|
|
cli_main_loop(ssh_sftp_pw_setup, ssh_sftp_pw_check,
|
|
ssh_sftp_mainloop_continue, ctx);
|
|
|
|
return ctx->toret;
|
|
}
|
|
|
|
/*
|
|
* Wait for some network data and process it.
|
|
*/
|
|
int ssh_sftp_loop_iteration(void)
|
|
{
|
|
return ssh_sftp_do_select(false, false);
|
|
}
|
|
|
|
/*
|
|
* Read a PSFTP command line from stdin.
|
|
*/
|
|
char *ssh_sftp_get_cmdline(const char *prompt, bool no_fds_ok)
|
|
{
|
|
char *buf;
|
|
size_t buflen, bufsize;
|
|
int ret;
|
|
|
|
fputs(prompt, stdout);
|
|
fflush(stdout);
|
|
|
|
buf = NULL;
|
|
buflen = bufsize = 0;
|
|
|
|
while (1) {
|
|
ret = ssh_sftp_do_select(true, no_fds_ok);
|
|
if (ret < 0) {
|
|
printf("connection died\n");
|
|
sfree(buf);
|
|
return NULL; /* woop woop */
|
|
}
|
|
if (ret > 0) {
|
|
sgrowarray(buf, bufsize, buflen);
|
|
ret = read(0, buf+buflen, 1);
|
|
if (ret < 0) {
|
|
perror("read");
|
|
sfree(buf);
|
|
return NULL;
|
|
}
|
|
if (ret == 0) {
|
|
/* eof on stdin; no error, but no answer either */
|
|
sfree(buf);
|
|
return NULL;
|
|
}
|
|
|
|
if (buf[buflen++] == '\n') {
|
|
/* we have a full line */
|
|
return buf;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
void frontend_net_error_pending(void) {}
|
|
|
|
void platform_psftp_pre_conn_setup(LogPolicy *lp) {}
|
|
|
|
const bool buildinfo_gtk_relevant = false;
|
|
|
|
/*
|
|
* Main program: do platform-specific initialisation and then call
|
|
* psftp_main().
|
|
*/
|
|
int main(int argc, char *argv[])
|
|
{
|
|
enable_dit();
|
|
uxsel_init();
|
|
CmdlineArgList *arglist = cmdline_arg_list_from_argv(argc, argv);
|
|
return psftp_main(arglist);
|
|
}
|