mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-01-09 09:27:59 +00:00
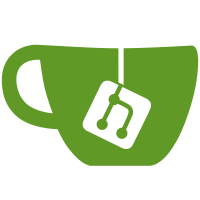
This gets rid of that awkward STANDARD_PREFIX system in which every branch of the old 'union control' had to repeat all the generic fields, and then call sites had to make an arbitrary decision about which branch to access them through. That was the best we could do before accepting C99 features in this code base. But now we have anonymous unions, so we don't need to put up with that nonsense any more! 'dlgcontrol' is now a struct rather than a union, and the generic fields common to all control types are ordinary members of the struct, so you don't have to refer to them as ctrl->generic.foo at all, just ctrl->foo, which saves verbiage at the point of use. The extra per-control fields are still held in structures named after the control type, so you'll still say ctrl->listbox.height or whatever. But now those structures are themselves members of an anonymous union field following the generic fields, so those sub-structures don't have to reiterate all the standard stuff too. While I'm here, I've promoted 'context2' from an editbox-specific field to a generic one (it just seems silly _not_ to allow any control to have two context fields if it needs it). Also, I had to rename the boolean field 'tabdelay' to avoid it clashing with the subsidiary structure field 'tabdelay', now that the former isn't generic.tabdelay any more.
50 lines
1.3 KiB
C
50 lines
1.3 KiB
C
/*
|
|
* config-unix.c - the Unix-specific parts of the PuTTY configuration
|
|
* box.
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <stdlib.h>
|
|
|
|
#include "putty.h"
|
|
#include "dialog.h"
|
|
#include "storage.h"
|
|
|
|
void unix_setup_config_box(struct controlbox *b, bool midsession, int protocol)
|
|
{
|
|
struct controlset *s;
|
|
dlgcontrol *c;
|
|
|
|
/*
|
|
* The Conf structure contains two Unix-specific elements which
|
|
* are not configured in here: stamp_utmp and login_shell. This
|
|
* is because pterm does not put up a configuration box right at
|
|
* the start, which is the only time when these elements would
|
|
* be useful to configure.
|
|
*/
|
|
|
|
/*
|
|
* On Unix, we don't have a drop-down list for the printer
|
|
* control.
|
|
*/
|
|
s = ctrl_getset(b, "Terminal", "printing", "Remote-controlled printing");
|
|
assert(s->ncontrols == 1 && s->ctrls[0]->type == CTRL_EDITBOX);
|
|
s->ctrls[0]->editbox.has_list = false;
|
|
|
|
/*
|
|
* Unix supports a local-command proxy.
|
|
*/
|
|
if (!midsession) {
|
|
int i;
|
|
s = ctrl_getset(b, "Connection/Proxy", "basics", NULL);
|
|
for (i = 0; i < s->ncontrols; i++) {
|
|
c = s->ctrls[i];
|
|
if (c->type == CTRL_LISTBOX &&
|
|
c->handler == proxy_type_handler) {
|
|
c->context.i |= PROXY_UI_FLAG_LOCAL;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|