mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-16 02:27:32 -05:00
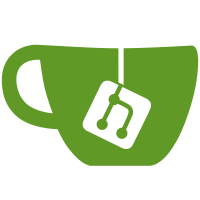
Mostly because I just had a neat idea about how to expose that large mutable array without it being a mutable global variable: make it a static in its own module, and expose only a _pointer_ to it, which is const-qualified. While I'm there, changed the name to something more descriptive.
45 lines
1.1 KiB
C
45 lines
1.1 KiB
C
/*
|
|
* smallprimes.c: implementation of the array of small primes defined
|
|
* in sshkeygen.h.
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include "ssh.h"
|
|
#include "sshkeygen.h"
|
|
|
|
/* The real array that stores the primes. It has to be writable in
|
|
* this module, but outside this module, we only expose the
|
|
* const-qualified pointer 'smallprimes' so that nobody else can
|
|
* accidentally overwrite it. */
|
|
static unsigned short smallprimes_array[NSMALLPRIMES];
|
|
|
|
const unsigned short *const smallprimes = smallprimes_array;
|
|
|
|
void init_smallprimes(void)
|
|
{
|
|
if (smallprimes_array[0])
|
|
return; /* already done */
|
|
|
|
bool A[65536];
|
|
|
|
for (size_t i = 2; i < lenof(A); i++)
|
|
A[i] = true;
|
|
|
|
for (size_t i = 2; i < lenof(A); i++) {
|
|
if (!A[i])
|
|
continue;
|
|
for (size_t j = 2*i; j < lenof(A); j += i)
|
|
A[j] = false;
|
|
}
|
|
|
|
size_t pos = 0;
|
|
for (size_t i = 2; i < lenof(A); i++) {
|
|
if (A[i]) {
|
|
assert(pos < NSMALLPRIMES);
|
|
smallprimes_array[pos++] = i;
|
|
}
|
|
}
|
|
|
|
assert(pos == NSMALLPRIMES);
|
|
}
|