mirror of
https://git.tartarus.org/simon/putty.git
synced 2025-07-12 00:33:53 -05:00
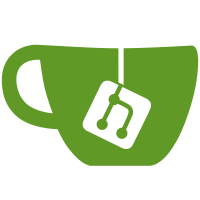
Windows Plink and PSFTP had very similar implementations, and now they share one that lives in a new file winselcli.c. I've similarly moved GUI PuTTY's implementation out of window.c into winselgui.c, where other GUI programs wanting to do networking will be able to access that too. In the spirit of centralisation, I've also taken the opportunity to make both functions use the reasonably complete winsock_error_string() rather than (for some historical reason) each inlining a minimal version that reports most errors as 'unknown'.
39 lines
794 B
C
39 lines
794 B
C
/*
|
|
* Implementation of do_select() for winnet.c to use, that uses
|
|
* WSAAsyncSelect to convert network activity into window messages,
|
|
* for integration into a GUI event loop.
|
|
*/
|
|
|
|
#include "putty.h"
|
|
|
|
static HWND winsel_hwnd = NULL;
|
|
|
|
void winselgui_set_hwnd(HWND hwnd)
|
|
{
|
|
winsel_hwnd = hwnd;
|
|
}
|
|
|
|
void winselgui_clear_hwnd(void)
|
|
{
|
|
winsel_hwnd = NULL;
|
|
}
|
|
|
|
char *do_select(SOCKET skt, bool enable)
|
|
{
|
|
int msg, events;
|
|
if (enable) {
|
|
msg = WM_NETEVENT;
|
|
events = (FD_CONNECT | FD_READ | FD_WRITE |
|
|
FD_OOB | FD_CLOSE | FD_ACCEPT);
|
|
} else {
|
|
msg = events = 0;
|
|
}
|
|
|
|
assert(winsel_hwnd);
|
|
|
|
if (p_WSAAsyncSelect(skt, winsel_hwnd, msg, events) == SOCKET_ERROR)
|
|
return winsock_error_string(p_WSAGetLastError());
|
|
|
|
return NULL;
|
|
}
|